Demo 40 of 68 in category RedBook
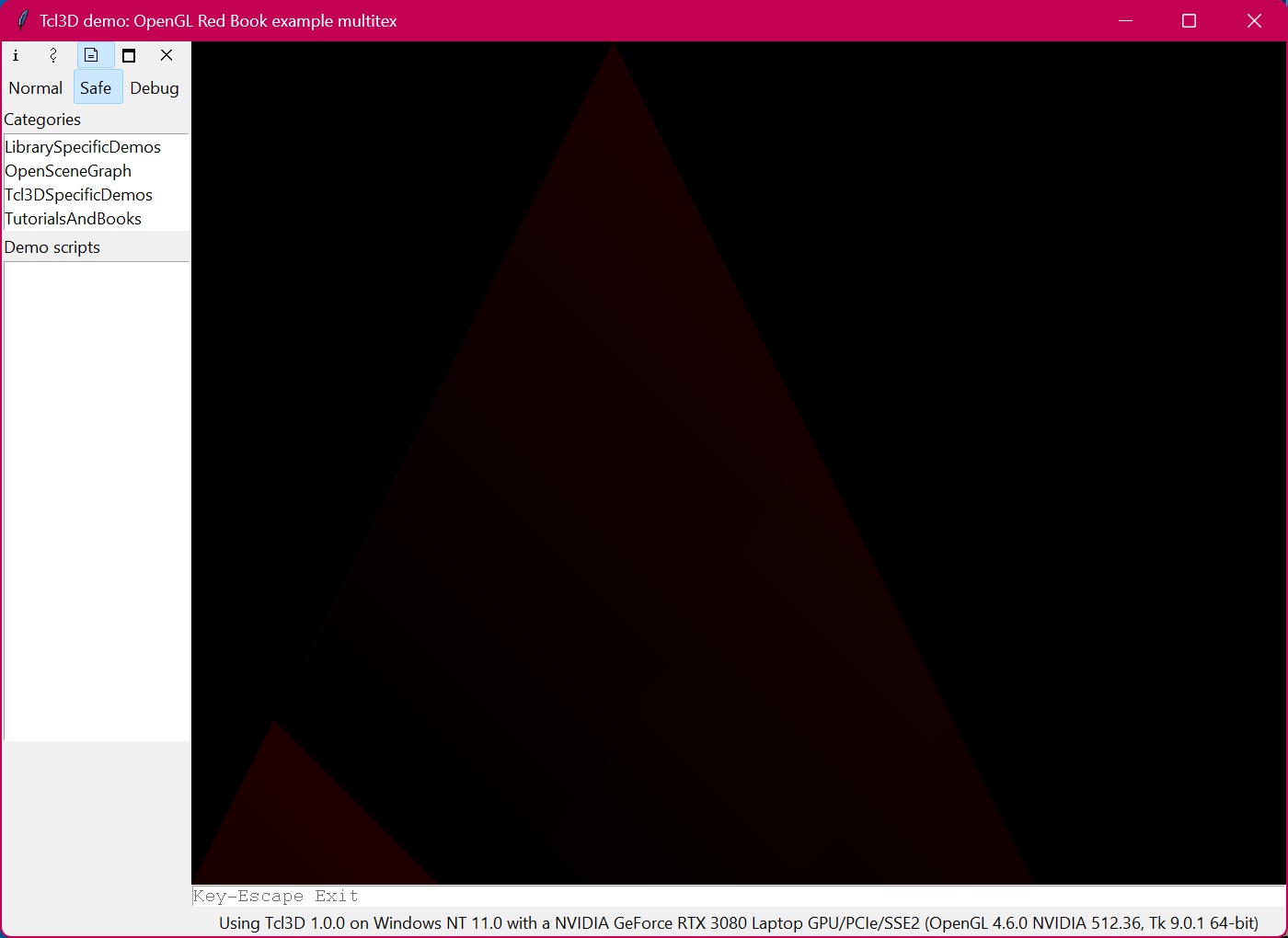 |
# multitex.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2025, Paul Obermeier.
# See file LICENSE for complete license information.
package require tcl3d
set texels0 [tcl3dVector GLubyte [expr 32*32*4]]
set texels1 [tcl3dVector GLubyte [expr 16*16*4]]
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc min { a b } {
if { $a < $b } {
return $a
} else {
return $b
}
}
# Note: A faster method to calculate and specify textures in Tcl has been
# introduced with Tcl3D version 0.3. See Tcl3D demo bytearray.tcl.
proc makeCheckImages {} {
for { set i 0 } { $i < 32 } { incr i } {
for { set j 0 } { $j < 32 } { incr j } {
$::texels0 set [expr {($i*32 + $j)*4 + 0}] $i
$::texels0 set [expr {($i*32 + $j)*4 + 1}] $j
$::texels0 set [expr {($i*32 + $j)*4 + 2}] [min [expr ($i*$j)/255] 255]
$::texels0 set [expr {($i*32 + $j)*4 + 3}] 255
}
}
for { set i 0 } { $i < 16 } { incr i } {
for { set j 0 } { $j < 16 } { incr j } {
$::texels1 set [expr {($i*16 + $j)*4 + 0}] 255
$::texels1 set [expr {($i*16 + $j)*4 + 1}] $i
$::texels1 set [expr {($i*16 + $j)*4 + 2}] $j
$::texels1 set [expr {($i*16 + $j)*4 + 3}] 255
}
}
}
proc CreateCallback { toglwin } {
set ::texNames [tcl3dVector GLuint 2]
glClearColor 0.0 0.0 0.0 0.0
glShadeModel GL_FLAT
glEnable GL_DEPTH_TEST
makeCheckImages
glPixelStorei GL_UNPACK_ALIGNMENT 1
glGenTextures 2 $::texNames
glBindTexture GL_TEXTURE_2D [$::texNames get 0]
glTexImage2D GL_TEXTURE_2D 0 $::GL_RGBA 32 32 0 GL_RGBA \
GL_UNSIGNED_BYTE $::texels0
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MAG_FILTER $::GL_NEAREST
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MIN_FILTER $::GL_NEAREST
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_WRAP_S $::GL_REPEAT
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_WRAP_T $::GL_REPEAT
glBindTexture GL_TEXTURE_2D [$::texNames get 1]
glTexImage2D GL_TEXTURE_2D 0 $::GL_RGBA 16 16 0 GL_RGBA \
GL_UNSIGNED_BYTE $::texels1
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MAG_FILTER $::GL_LINEAR
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MIN_FILTER $::GL_LINEAR
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_WRAP_S $::GL_CLAMP_TO_EDGE
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_WRAP_T $::GL_CLAMP_TO_EDGE
# Use the two texture objects to define two texture units
# for use in multitexturing.
glActiveTexture GL_TEXTURE0
glEnable GL_TEXTURE_2D
glBindTexture GL_TEXTURE_2D [$::texNames get 0]
glTexEnvi GL_TEXTURE_ENV GL_TEXTURE_ENV_MODE $::GL_REPLACE
glMatrixMode GL_TEXTURE
glLoadIdentity
glTranslatef 0.5 0.5 0.0
glRotatef 45.0 0.0 0.0 1.0
glTranslatef -0.5 -0.5 0.0
glMatrixMode GL_MODELVIEW
glActiveTexture GL_TEXTURE1
glEnable GL_TEXTURE_2D
glBindTexture GL_TEXTURE_2D [$::texNames get 1]
glTexEnvi GL_TEXTURE_ENV GL_TEXTURE_ENV_MODE $::GL_MODULATE
}
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glBegin GL_TRIANGLES
glMultiTexCoord2f GL_TEXTURE0 0.0 0.0
glMultiTexCoord2f GL_TEXTURE1 1.0 0.0
glVertex2f 0.0 0.0
glMultiTexCoord2f GL_TEXTURE0 0.5 1.0
glMultiTexCoord2f GL_TEXTURE1 0.5 0.0
glVertex2f 50.0 100.0
glMultiTexCoord2f GL_TEXTURE0 1.0 0.0
glMultiTexCoord2f GL_TEXTURE1 1.0 1.0
glVertex2f 100.0 0.0
glEnd
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
if { $w <= $h } {
gluOrtho2D 0.0 100.0 0.0 [expr 100.0 * double($h)/double($w)]
} else {
gluOrtho2D 0.0 [expr 100.0 * double($w)/double($h)] 0.0 100.0
}
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 250 -height 250 -double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 1
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example multitex"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
if { ! [tcl3dOglHaveVersion 1 2] } {
tk_messageBox -icon warning -type ok -title "Invalid OpenGL version" \
-message [format "Feature needs OpenGL >= 1.2. Only have %s" \
[glGetString GL_VERSION]]
}
|
