Demo 39 of 68 in category RedBook
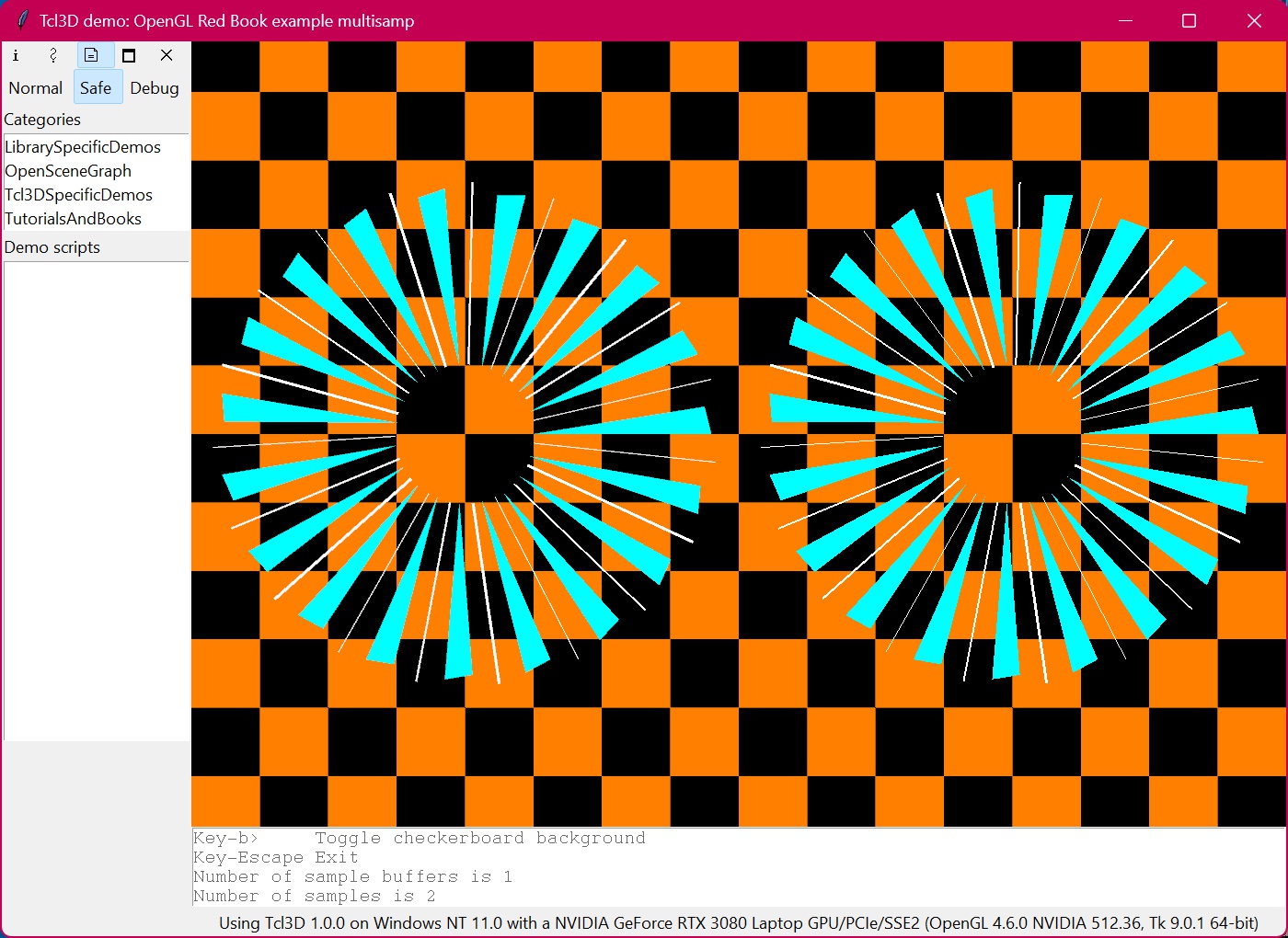 |
# multisamp.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program draws shows how to use multisampling to
# draw anti-aliased geometric primitives. The same
# display list, a pinwheel of triangles and lines of
# varying widths, is rendered twice. Multisampling is
# enabled when the left side is drawn. Multisampling is
# disabled when the right side is drawn.
#
# Pressing the 'b' key toggles drawing of the checkerboard
# background. Antialiasing is sometimes easier to see
# when objects are rendered over a contrasting background.
#
# This demo uses the multisampling options built into tcl3dTogl starting
# from version 0.3.2.
# Another way to set the number of samples is via the driver specific GUI under
# Windows, or by setting the environment variable __GL_FSAA_MODE under Linux.
package require tcl3d
set bgtoggle 1
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
#
# Print out state values related to multisampling.
# Create display list with "pinwheel" of lines and
# triangles.
proc CreateCallback { toglwin } {
glClearColor 0.0 0.0 0.0 0.0
glNewList 1 GL_COMPILE
for { set i 0 } { $i < 19 } { incr i } {
glPushMatrix
glRotatef [expr 360.0*double($i)/19.0] 0.0 0.0 1.0
glColor3f 1.0 1.0 1.0
glLineWidth [expr ($i%3)+1.0]
glBegin GL_LINES
glVertex2f 0.25 0.05
glVertex2f 0.9 0.2
glEnd
glColor3f 0.0 1.0 1.0
glBegin GL_TRIANGLES
glVertex2f 0.25 0.0
glVertex2f 0.9 0.0
glVertex2f 0.875 0.10
glEnd
glPopMatrix
}
glEndList
glNewList 2 GL_COMPILE
glColor3f 1.0 0.5 0.0
glBegin GL_QUADS
for { set i 0 } { $i < 16 } { incr i } {
for { set j 0 } { $j < 16 } { incr j } {
if { ((($i + $j) % 2) == 0) } {
glVertex2f [expr -2.0 + $i * 0.25] [expr -2.0 + $j * 0.25]
glVertex2f [expr -2.0 + $i * 0.25] [expr -1.75 + $j * 0.25]
glVertex2f [expr -1.75 + $i * 0.25] [expr -1.75 + $j * 0.25]
glVertex2f [expr -1.75 + $i * 0.25] [expr -2.0 + $j * 0.25]
}
}
}
glEnd
glEndList
}
# Draw two sets of primitives, so that you can
# compare the user of multisampling against its absence.
#
# This code enables antialiasing and draws one display list
# and disables and draws the other display list
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
if { $::bgtoggle } {
glCallList 2
}
glEnable GL_MULTISAMPLE
glPushMatrix
glTranslatef -1.0 0.0 0.0
glCallList 1
glPopMatrix
glDisable GL_MULTISAMPLE
glPushMatrix
glTranslatef 1.0 0.0 0.0
glCallList 1
glPopMatrix
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
if { $w <= (2 * $h) } {
gluOrtho2D -2.0 2.0 [expr -2.0*double($h)/double($w)] \
[expr 2.0*double($h)/double($w)]
} else {
gluOrtho2D [expr -2.0*double($w)/double($h)] \
[expr 2.0*double($w)/double($h)] -2.0 2.0
}
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
proc toggle { toglwin } {
set ::bgtoggle [expr !$::bgtoggle]
$toglwin postredisplay
}
frame .fr
pack .fr -expand 1 -fill both
# Create a Togl window using multisample buffers.
# Reshape and Display callbacks are configured later after knowing if
# the needed multisample buffers are available.
set retVal [catch { togl .fr.toglwin \
-width 600 -height 500 -double true \
-multisamplebuffers 1 -multisamplesamples 2 \
-createcommand CreateCallback } errMsg]
if { $retVal != 0 } {
tk_messageBox -icon error -type ok -title "Missing Togl feature" \
-message "Demo needs multisample buffers: $errMsg"
proc ::Cleanup {} {}
exit 1
return
}
.fr.toglwin configure \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 4
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example multisamp"
bind . <Key-b> "toggle .fr.toglwin"
bind . <Key-Escape> "exit"
set sbufs [tcl3dOglGetIntegerState GL_SAMPLE_BUFFERS]
set samples [tcl3dOglGetIntegerState GL_SAMPLES]
.fr.usage insert end "Key-b> Toggle checkerboard background"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Number of sample buffers is $sbufs"
.fr.usage insert end "Number of samples is $samples"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
if { ! [tcl3dOglHaveVersion 1 3] } {
tk_messageBox -icon warning -type ok -title "Invalid OpenGL version" \
-message [format "Feature needs OpenGL >= 1.3. Only have %s" \
[glGetString GL_VERSION]]
}
|
