Demo 26 of 68 in category RedBook
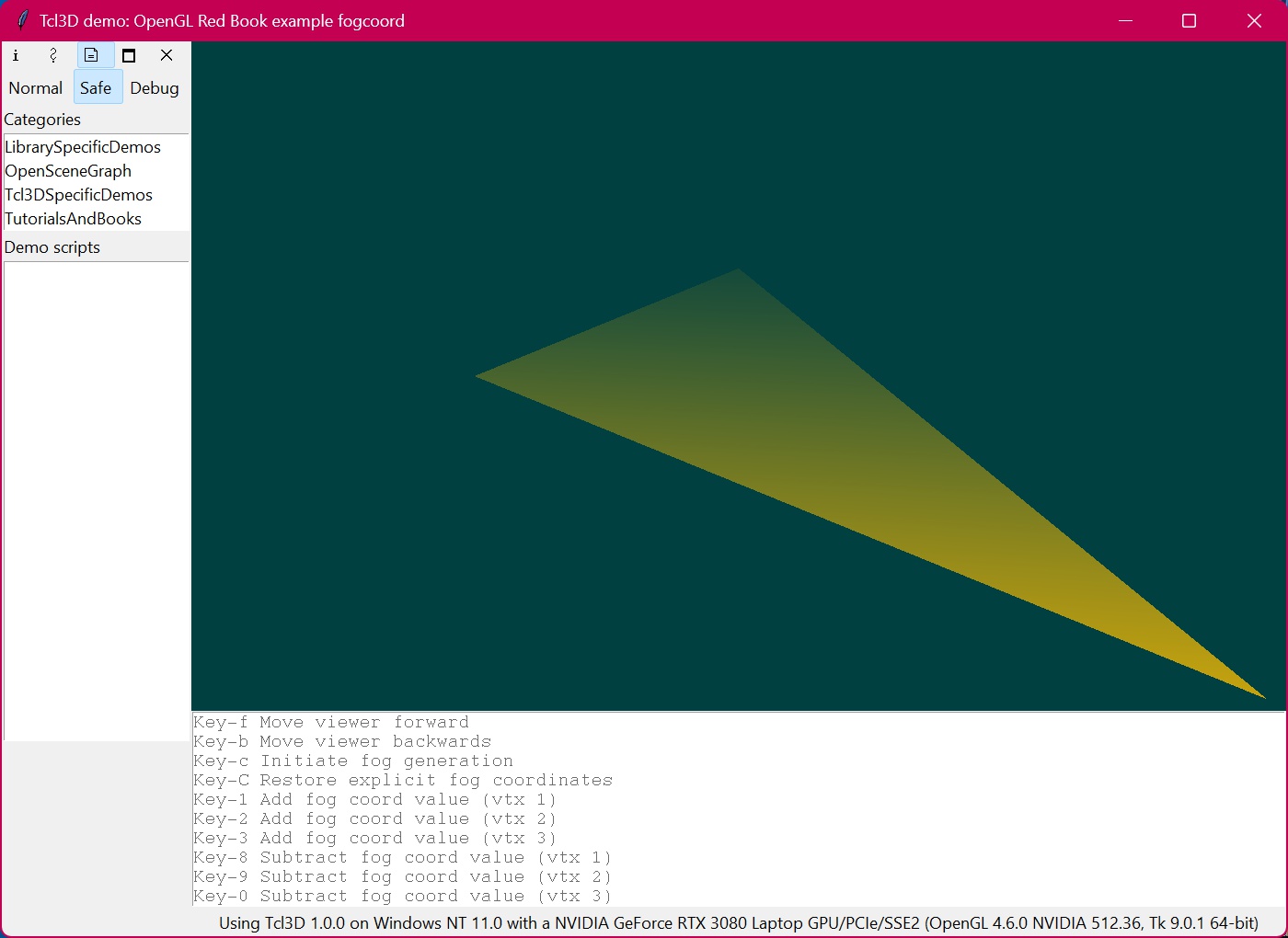 |
# fogcoord.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2025, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program demonstrates the use of explicit fog
# coordinates. You can press the keyboard and change
# the fog coordinate value at any vertex. You can
# also switch between using explicit fog coordinates
# and the default fog generation mode.
#
# Pressing the 'f' and 'b' keys move the viewer forward
# and backwards.
# Pressing 'c' initiates the default fog generation.
# Pressing capital 'C' restores explicit fog coordinates.
# Pressing '1', '2', '3', '8', '9', and '0' add or
# subtract from the fog coordinate values at one of the
# three vertices of the triangle.
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
global g_Fog
set fogColor {0.0 0.25 0.25 1.0}
set g_Fog(1) 1.0
set g_Fog(2) 5.0
set g_Fog(3) 10.0
glEnable GL_FOG
glFogi GL_FOG_MODE $::GL_EXP
glFogfv GL_FOG_COLOR $fogColor
glFogf GL_FOG_DENSITY 0.25
glHint GL_FOG_HINT GL_DONT_CARE
glFogi GL_FOG_COORDINATE_SOURCE $::GL_FOG_COORDINATE
glClearColor 0.0 0.25 0.25 1.0 ; # fog color
}
# display draws a triangle at an angle.
#
proc DisplayCallback { toglwin } {
global g_Fog
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glColor3f 1.0 0.75 0.0
glBegin GL_TRIANGLES
glFogCoordf $g_Fog(1)
glVertex3f 2.0 -2.0 0.0
glFogCoordf $g_Fog(2)
glVertex3f -2.0 0.0 -5.0
glFogCoordf $g_Fog(3)
glVertex3f 0.0 2.0 -10.0
glEnd
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 1.0 0.25 25.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
glTranslatef 0.0 0.0 -5.0
}
proc key_c {} {
glFogi GL_FOG_COORDINATE_SOURCE $::GL_FRAGMENT_DEPTH
.fr.toglwin postredisplay
}
proc key_C {} {
glFogi GL_FOG_COORDINATE_SOURCE $::GL_FOG_COORDINATE
.fr.toglwin postredisplay
}
proc key_1 {} {
global g_Fog
set g_Fog(1) [expr $g_Fog(1) + 0.25]
.fr.toglwin postredisplay
}
proc key_2 {} {
global g_Fog
set g_Fog(2) [expr $g_Fog(2) + 0.25]
.fr.toglwin postredisplay
}
proc key_3 {} {
global g_Fog
set g_Fog(3) [expr $g_Fog(3) + 0.25]
.fr.toglwin postredisplay
}
proc key_8 {} {
global g_Fog
if { $g_Fog(1) > 0.25 } {
set g_Fog(1) [expr $g_Fog(1) - 0.25]
}
.fr.toglwin postredisplay
}
proc key_9 {} {
global g_Fog
if { $g_Fog(2) > 0.25 } {
set g_Fog(2) [expr $g_Fog(2) - 0.25]
}
.fr.toglwin postredisplay
}
proc key_0 {} {
global g_Fog
if { $g_Fog(3) > 0.25 } {
set g_Fog(3) [expr $g_Fog(3) - 0.25]
}
.fr.toglwin postredisplay
}
proc key_b {} {
glMatrixMode GL_MODELVIEW
glTranslatef 0.0 0.0 -0.25
.fr.toglwin postredisplay
}
proc key_f {} {
glMatrixMode GL_MODELVIEW
glTranslatef 0.0 0.0 0.25
.fr.toglwin postredisplay
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 10
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example fogcoord"
bind . <Key-f> key_f
bind . <Key-b> key_b
bind . <Key-c> key_c
bind . <Key-C> key_C
bind . <Key-1> key_1
bind . <Key-2> key_2
bind . <Key-3> key_3
bind . <Key-8> key_8
bind . <Key-9> key_9
bind . <Key-0> key_0
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-f Move viewer forward"
.fr.usage insert end "Key-b Move viewer backwards"
.fr.usage insert end "Key-c Initiate fog generation"
.fr.usage insert end "Key-C Restore explicit fog coordinates"
.fr.usage insert end "Key-1 Add fog coord value (vtx 1)"
.fr.usage insert end "Key-2 Add fog coord value (vtx 2)"
.fr.usage insert end "Key-3 Add fog coord value (vtx 3)"
.fr.usage insert end "Key-8 Subtract fog coord value (vtx 1)"
.fr.usage insert end "Key-9 Subtract fog coord value (vtx 2)"
.fr.usage insert end "Key-0 Subtract fog coord value (vtx 3)"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
if { ! [tcl3dOglHaveVersion 1 4] } {
tk_messageBox -icon warning -type ok -title "Invalid OpenGL version" \
-message [format "Feature needs OpenGL >= 1.4. Only have %s" \
[glGetString GL_VERSION]]
}
|
