Demo 25 of 68 in category RedBook
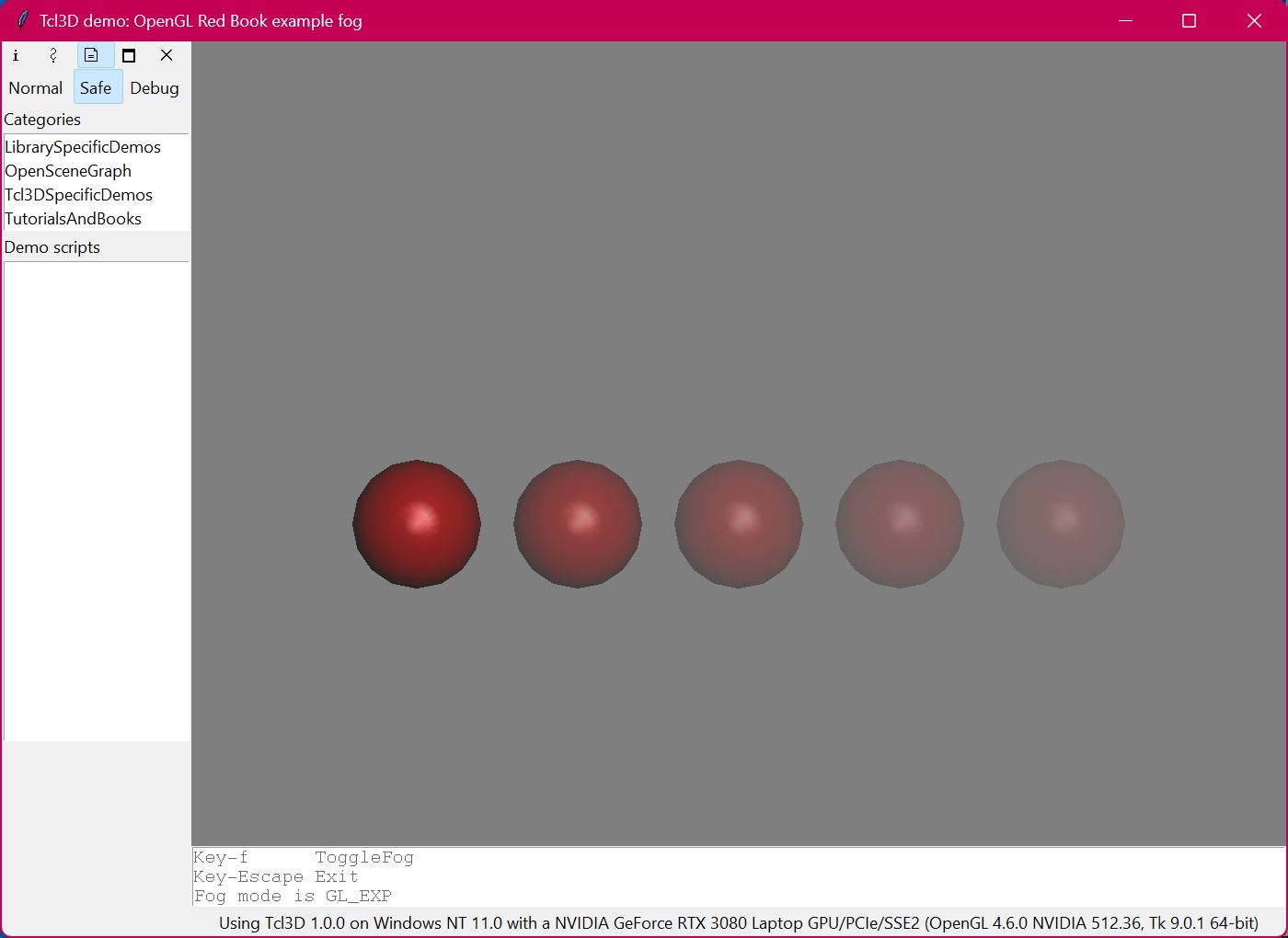 |
# fog.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2025, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program draws 5 red spheres, each at a different
# z distance from the eye, in different types of fog.
# Pressing the f key chooses between 3 types of
# fog: exponential, exponential squared, and linear.
# In this program, there is a fixed density value, as well
# as fixed start and end values for the linear fog.
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
# Initialize depth buffer, fog, light source,
# material property, and lighting model.
proc CreateCallback { toglwin } {
set position { 0.5 0.5 3.0 0.0 }
glEnable GL_DEPTH_TEST
glLightfv GL_LIGHT0 GL_POSITION $position
glEnable GL_LIGHTING
glEnable GL_LIGHT0
set mat {0.1745 0.01175 0.01175}
glMaterialfv GL_FRONT GL_AMBIENT $mat
set mat { 0.61424 0.04136 0.04136 }
glMaterialfv GL_FRONT GL_DIFFUSE $mat
set mat { 0.727811 0.626959 0.626959 }
glMaterialfv GL_FRONT GL_SPECULAR $mat
glMaterialf GL_FRONT GL_SHININESS [expr 0.6*128.0]
glEnable GL_FOG
set fogColor {0.5 0.5 0.5 1.0}
glFogi GL_FOG_MODE $::fogMode
glFogfv GL_FOG_COLOR $fogColor
glFogf GL_FOG_DENSITY 0.35
glHint GL_FOG_HINT GL_DONT_CARE
glFogf GL_FOG_START 1.0
glFogf GL_FOG_END 5.0
glClearColor 0.5 0.5 0.5 1.0 ; # fog color
}
proc renderSphere { x y z } {
glPushMatrix
glTranslatef $x $y $z
glutSolidSphere 0.4 16 16
glPopMatrix
}
# display() draws 5 spheres at different z positions.
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
renderSphere -2.0 -0.5 -1.0
renderSphere -1.0 -0.5 -2.0
renderSphere 0.0 -0.5 -3.0
renderSphere 1.0 -0.5 -4.0
renderSphere 2.0 -0.5 -5.0
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
if { $w <= $h } {
glOrtho -2.5 2.5 \
[expr -2.5*double($h)/double($w)] \
[expr 2.5*double($h)/double($w)] \
-10.0 10.0
} else {
glOrtho [expr -2.5*double($w)/double($h)] \
[expr 2.5*double($w)/double($h)] \
-2.5 2.5 -10.0 10.0
}
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
proc UpdateInfo { fog } {
.fr.usage configure -state normal
.fr.usage delete end
.fr.usage insert end "Fog mode is $fog"
.fr.usage configure -state disabled
}
proc ToggleFog {} {
global fogMode
if { $fogMode == $::GL_EXP } {
set fogMode $::GL_EXP2
UpdateInfo "GL_EXP2"
} elseif { $fogMode == $::GL_EXP2 } {
set fogMode $::GL_LINEAR
UpdateInfo "GL_LINEAR"
} elseif { $fogMode == $::GL_LINEAR } {
set fogMode $::GL_EXP
UpdateInfo "GL_EXP"
}
glFogi GL_FOG_MODE $fogMode
.fr.toglwin postredisplay
}
set ::fogMode $::GL_EXP
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 3
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example fog"
bind . <Key-f> "ToggleFog"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-f ToggleFog"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Status line"
.fr.usage configure -state disabled
UpdateInfo "GL_EXP"
PrintInfo [tcl3dOglGetInfoString]
|
