Demo 15 of 68 in category RedBook
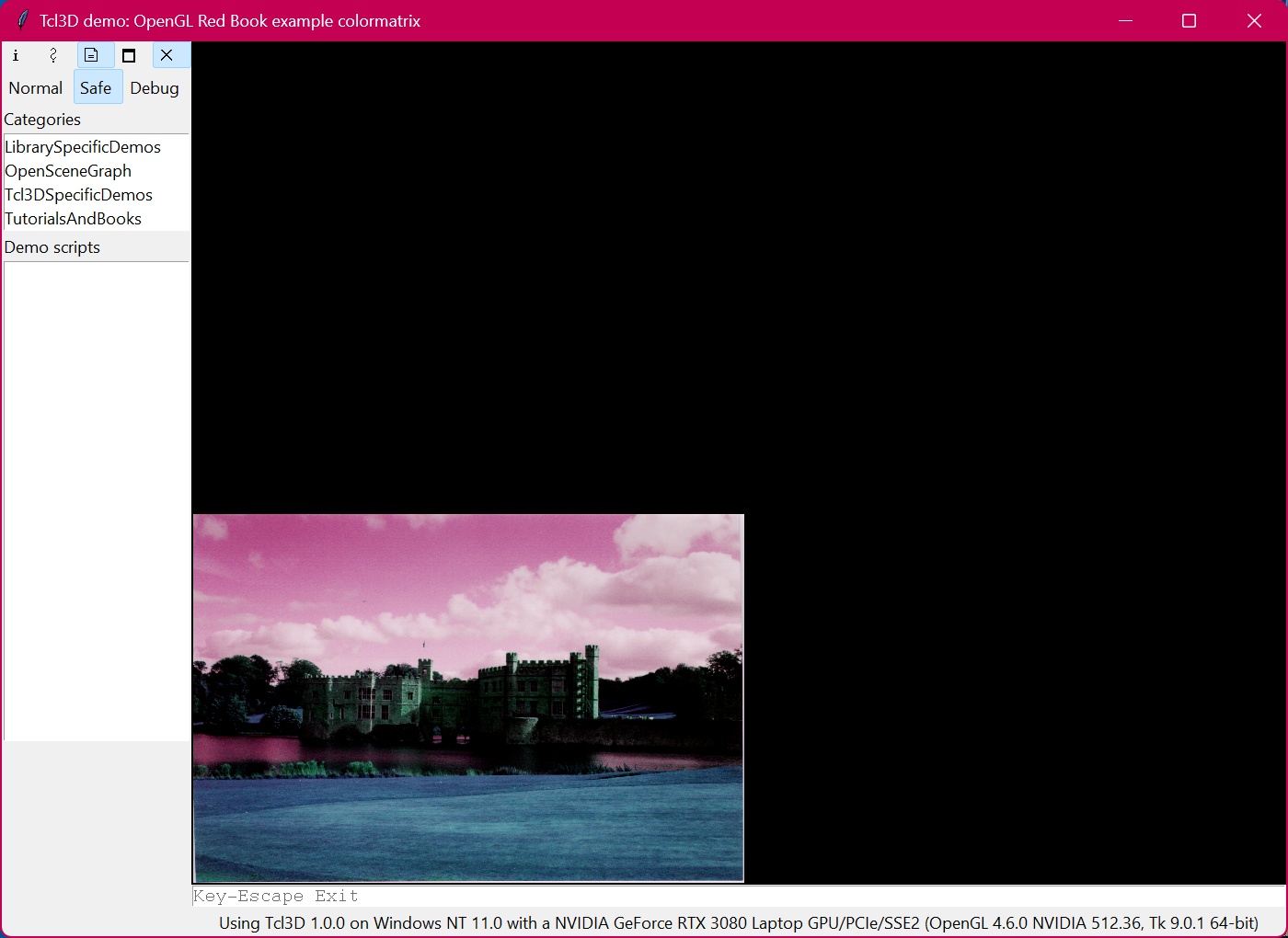 |
# colormatix.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program uses the color matrix to exchange the color channels of
# an image.
#
# Red -> Green
# Green -> Blue
# Blue -> Red
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
if { ! [tcl3dOglHaveExtension $toglwin "GL_ARB_imaging"] } {
tk_messageBox -icon error -type ok -title "Missing OpenGL extension" \
-message "Demo needs the GL_ARB_imaging extension."
proc ::Cleanup {} {}
exit 1
return
}
set m { 0.0 1.0 0.0 0.0
0.0 0.0 1.0 0.0
1.0 0.0 0.0 0.0
0.0 0.0 0.0 1.0 }
glPixelStorei GL_UNPACK_ALIGNMENT 1
glClearColor 0.0 0.0 0.0 0.0
glMatrixMode GL_COLOR
glLoadMatrixf $m
glMatrixMode GL_MODELVIEW
}
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glRasterPos2i 1 1
glDrawPixels $::width $::height GL_RGB GL_UNSIGNED_BYTE $::pixels
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
glOrtho 0 $::width 0 $::height -1.0 1.0
glMatrixMode GL_MODELVIEW
}
set widthVec [tcl3dVector GLsizei 1]
set heightVec [tcl3dVector GLsizei 1]
set imgName [file join [file dirname [info script]] "Data" "leeds.bin"]
set pixels [tcl3dReadRedBookImage [tcl3dGetExtFile $imgName] $widthVec $heightVec]
set width [$widthVec get 0]
set height [$heightVec get 0]
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width $width -height $height \
-double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 1
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example colormatrix"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
