Demo 9 of 9 in category GameProgrammer
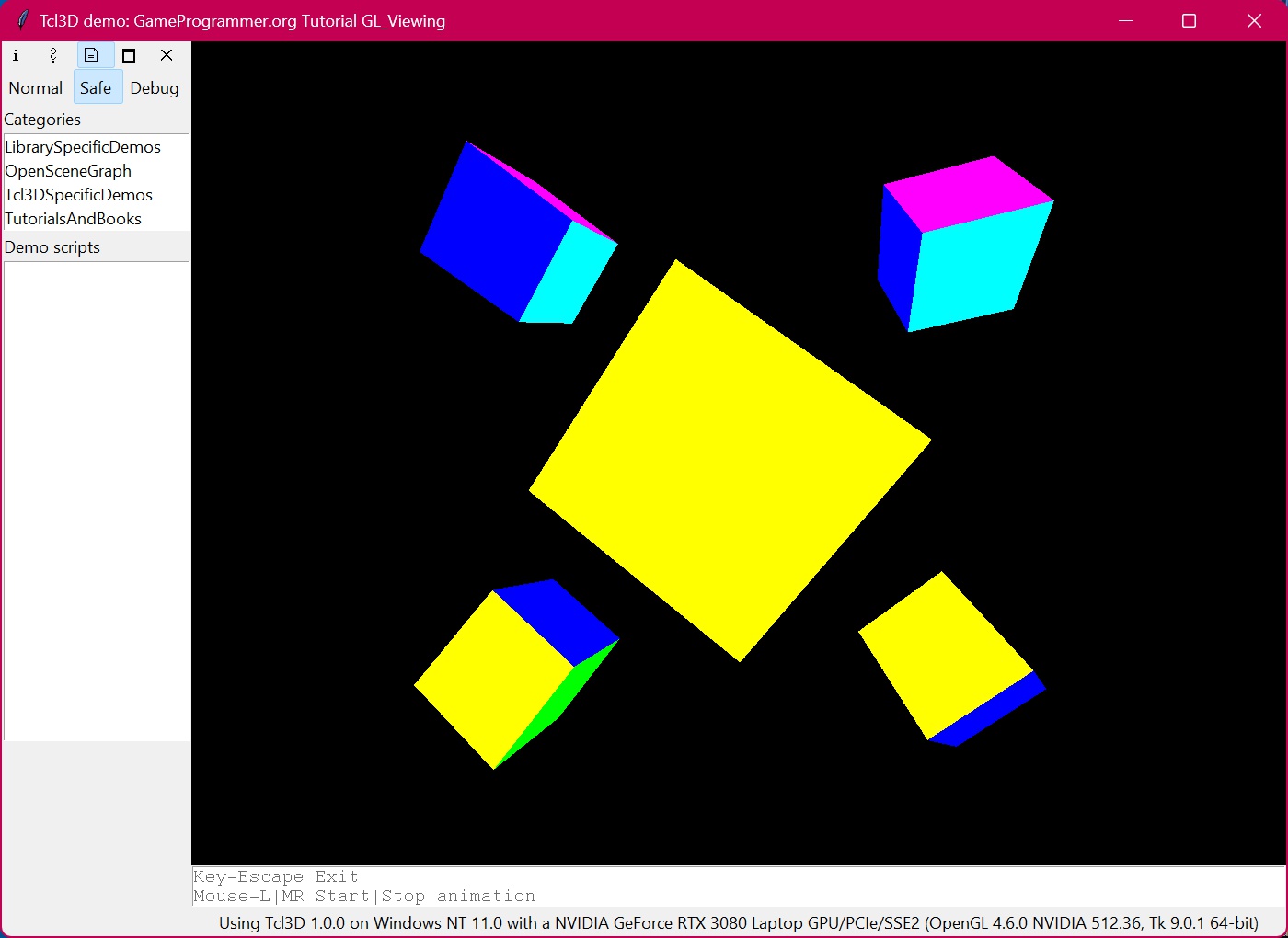 |
# GL_Viewing.tcl
#
# Tutorial from www.GameProgrammer.org
# Viewing and Transformations.
#
# Original code Copyright 2004 by Vahid Kazemi
#
# Modified for Tcl3D by Paul Obermeier 2006/09/11
# See www.tcl3d.org for the Tcl3D extension.
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Window size.
set winWidth 640
set winHeight 480
set rot 0.0
set rotIncr 1.0
set scl 1.0
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
ExitProg
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc DrawCube {} {
# Colorful Quad
glBegin GL_QUAD_STRIP
glColor3f 1 0 0
glVertex3f -0.5 -0.5 -0.5
glVertex3f 0.5 -0.5 -0.5
glColor3f 1 1 0
glVertex3f -0.5 0.5 -0.5
glVertex3f 0.5 0.5 -0.5
glColor3f 0 1 0
glVertex3f -0.5 0.5 0.5
glVertex3f 0.5 0.5 0.5
glColor3f 0 1 1
glVertex3f -0.5 -0.5 0.5
glVertex3f 0.5 -0.5 0.5
glColor3f 1 0 1
glVertex3f -0.5 -0.5 -0.5
glVertex3f 0.5 -0.5 -0.5
glEnd
glColor3f 0 0 1
glBegin GL_QUADS
glVertex3f -0.5 -0.5 -0.5
glVertex3f -0.5 0.5 -0.5
glVertex3f -0.5 0.5 0.5
glVertex3f -0.5 -0.5 0.5
glVertex3f 0.5 -0.5 -0.5
glVertex3f 0.5 0.5 -0.5
glVertex3f 0.5 0.5 0.5
glVertex3f 0.5 -0.5 0.5
glEnd
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 90.0 1.3 0.1 1000.0
glMatrixMode GL_MODELVIEW
}
# The Togl callback function called when window is resized.
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
}
# The Togl callback function called when window is created.
proc CreateCallback { toglwin } {
glEnable GL_DEPTH_TEST
glShadeModel GL_FLAT
}
# The Togl callback function for rendering a frame.
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
if { [info exists ::animateId] } {
set ::rot [expr {$::rot + $::rotIncr}]
set ::scl [expr {sin ($::rot/100.0)+1.1}]
}
glLoadIdentity
glTranslatef 0 0 -4
glPushMatrix
glTranslatef -2 -2 0
glRotatef $::rot 1.0 0.5 0.0
DrawCube
glPopMatrix
glPushMatrix
glTranslatef 2 -2 0
glRotatef $::rot 1.0 -0.5 0.5
DrawCube
glPopMatrix
glPushMatrix
glTranslatef 2 2 0
glRotatef $::rot 0.0 0.5 -1.0
DrawCube
glPopMatrix
glPushMatrix
glTranslatef -2 2 0
glRotatef $::rot 1.0 -0.5 1.0
DrawCube
glPopMatrix
glPushMatrix
glScalef $::scl $::scl $::scl
glRotatef $::rot -0.5 1.0 0.0
DrawCube
glPopMatrix
$toglwin swapbuffers
}
# Put all exit related code here.
proc ExitProg {} {
exit
}
proc Animate {} {
.fr.toglwin postredisplay
set ::animateId [tcl3dAfterIdle Animate]
}
proc StartAnimation {} {
if { ! [info exists ::animateId] } {
Animate
}
}
proc StopAnimation {} {
if { [info exists ::animateId] } {
after cancel $::animateId
unset ::animateId
}
}
# Create the OpenGL window and some Tk helper widgets.
proc CreateWindow {} {
frame .fr
pack .fr -expand 1 -fill both
# Create Our OpenGL Window
togl .fr.toglwin -width $::winWidth -height $::winHeight \
-swapinterval 1 \
-double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 2
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: GameProgrammer.org Tutorial GL_Viewing"
# Watch For ESC Key And Quit Messages
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
bind .fr.toglwin <1> "StartAnimation"
bind .fr.toglwin <2> "StopAnimation"
bind .fr.toglwin <3> "StopAnimation"
bind .fr.toglwin <Control-Button-1> "StopAnimation"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Mouse-L|MR Start|Stop animation"
.fr.usage configure -state disabled
}
CreateWindow
PrintInfo [tcl3dOglGetInfoString]
if { [file tail [info script]] eq [file tail $::argv0] } {
# If started directly from tclsh or wish, then start animation.
update
StartAnimation
}
|
