Demo 1 of 9 in category GameProgrammer
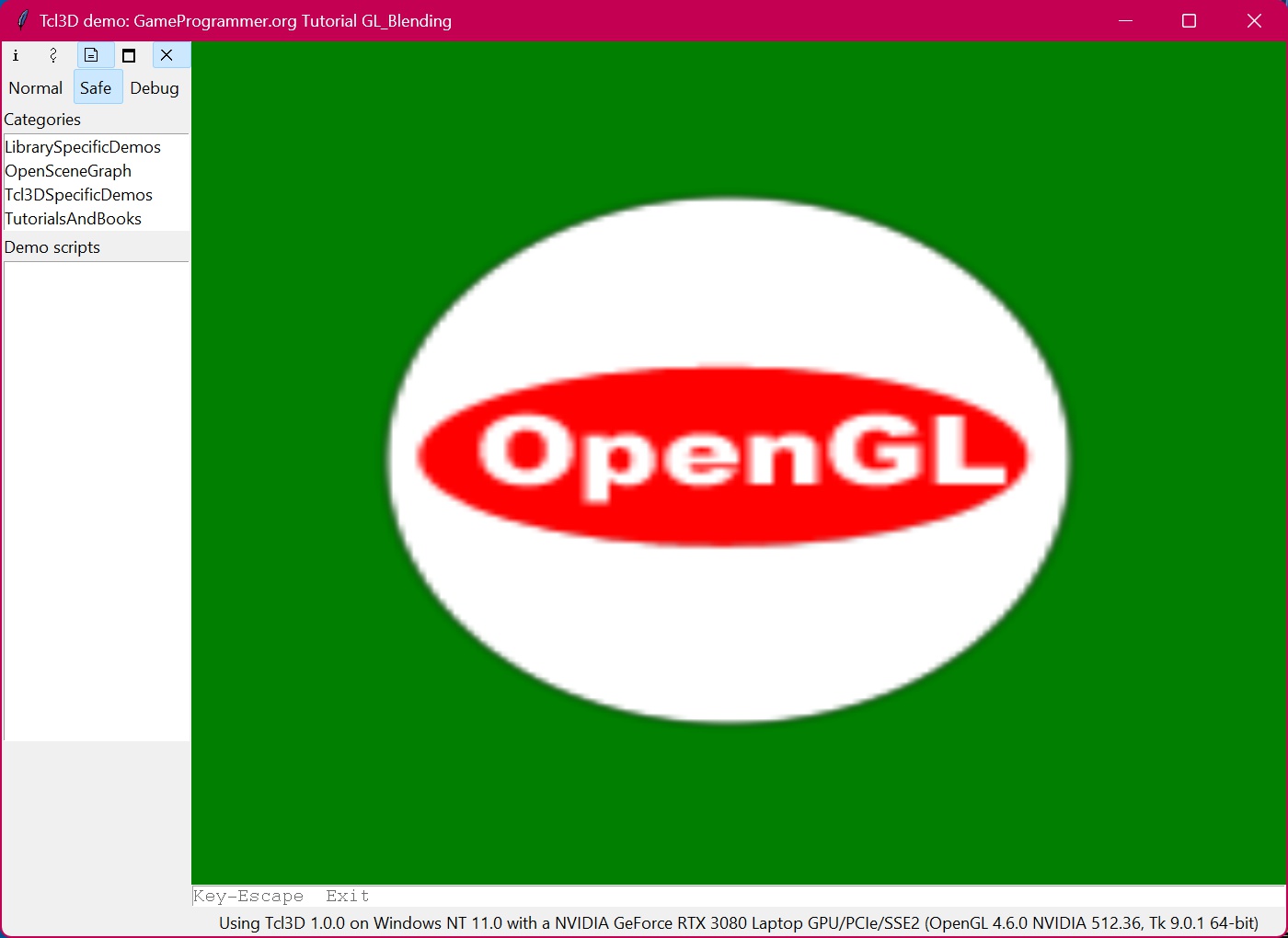 |
# GL_Blending.tcl
#
# Tutorial from www.GameProgrammer.org
# Blending demo
#
# Original code Copyright 2005 by Vahid Kazemi
#
# Modified for Tcl3D by Paul Obermeier 2006/09/12
# See www.tcl3d.org for the Tcl3D extension.
package require Img
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Determine the directory of this script.
set g_scriptDir [file dirname [info script]]
# Window size.
set winWidth 640
set winHeight 480
# Storage for 1 texture
set texId [tcl3dVector GLuint 1]
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
ExitProg
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc LoadImage { imgName numChans } {
if { $numChans != 3 && $numChans != 4 } {
error "Error: Only 3 or 4 channels allowed ($numChans supplied)"
}
set texName [file join $::g_scriptDir $imgName]
set retVal [catch {set phImg [image create photo -file $texName]} err1]
if { $retVal != 0 } {
error "Error reading image $texName ($err1)"
} else {
set w [image width $phImg]
set h [image height $phImg]
set texImg [tcl3dVectorFromPhoto $phImg $numChans]
image delete $phImg
}
return [list $texImg $w $h]
}
proc LoadTextureWithAlphaMask { imgName maskName } {
set imgInfo [LoadImage $maskName 3]
set imgData [lindex $imgInfo 0]
set imgWidth [lindex $imgInfo 1]
set imgHeight [lindex $imgInfo 2]
set finalImg [tcl3dVector GLubyte [expr $imgWidth * $imgHeight * 4]]
# Copy red channel (0) into alpha channel (3) of finalImg
tcl3dVectorCopyChannel $imgData $finalImg 0 3 $imgWidth $imgHeight 3 4
$imgData delete
set imgInfo [LoadImage $imgName 3]
set imgData [lindex $imgInfo 0]
# Copy red/green/blue channels into corresponding channels of finalImg
tcl3dVectorCopyChannel $imgData $finalImg 0 0 $imgWidth $imgHeight 3 4
tcl3dVectorCopyChannel $imgData $finalImg 1 1 $imgWidth $imgHeight 3 4
tcl3dVectorCopyChannel $imgData $finalImg 2 2 $imgWidth $imgHeight 3 4
$imgData delete
glGenTextures 1 $::texId
glBindTexture GL_TEXTURE_2D [$::texId get 0]
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MIN_FILTER $::GL_LINEAR
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MAG_FILTER $::GL_LINEAR
glTexImage2D GL_TEXTURE_2D 0 $::GL_RGBA $imgWidth $imgHeight \
0 GL_RGBA GL_UNSIGNED_BYTE $finalImg
$finalImg delete
}
# The Togl callback function called when window is resized.
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
}
# The Togl callback function called when window is created.
proc CreateCallback { toglwin } {
glEnable GL_BLEND
glBlendFunc GL_SRC_ALPHA GL_ONE_MINUS_SRC_ALPHA
glEnable GL_TEXTURE_2D
LoadTextureWithAlphaMask "texture.bmp" "mask.bmp"
glClearColor 0 0.5 0.0 1
}
# The Togl callback function for rendering a frame.
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glColor3f 1 1 1
glBindTexture GL_TEXTURE_2D [$::texId get 0]
glBegin GL_QUADS
glTexCoord2f 0 0 ; glVertex2f -0.8 -0.8
glTexCoord2f 1 0 ; glVertex2f 0.8 -0.8
glTexCoord2f 1 1 ; glVertex2f 0.8 0.8
glTexCoord2f 0 1 ; glVertex2f -0.8 0.8
glEnd
glFlush
$toglwin swapbuffers
}
# Put all exit related code here.
proc ExitProg {} {
exit
}
# Create the OpenGL window and some Tk helper widgets.
proc CreateWindow {} {
frame .fr
pack .fr -expand 1 -fill both
# Create Our OpenGL Window
togl .fr.toglwin -width $::winWidth -height $::winHeight \
-double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 1
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: GameProgrammer.org Tutorial GL_Blending"
# Watch For ESC Key And Quit Messages
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
}
CreateWindow
PrintInfo [tcl3dOglGetInfoString]
|
