Demo 6 of 9 in category GameProgrammer
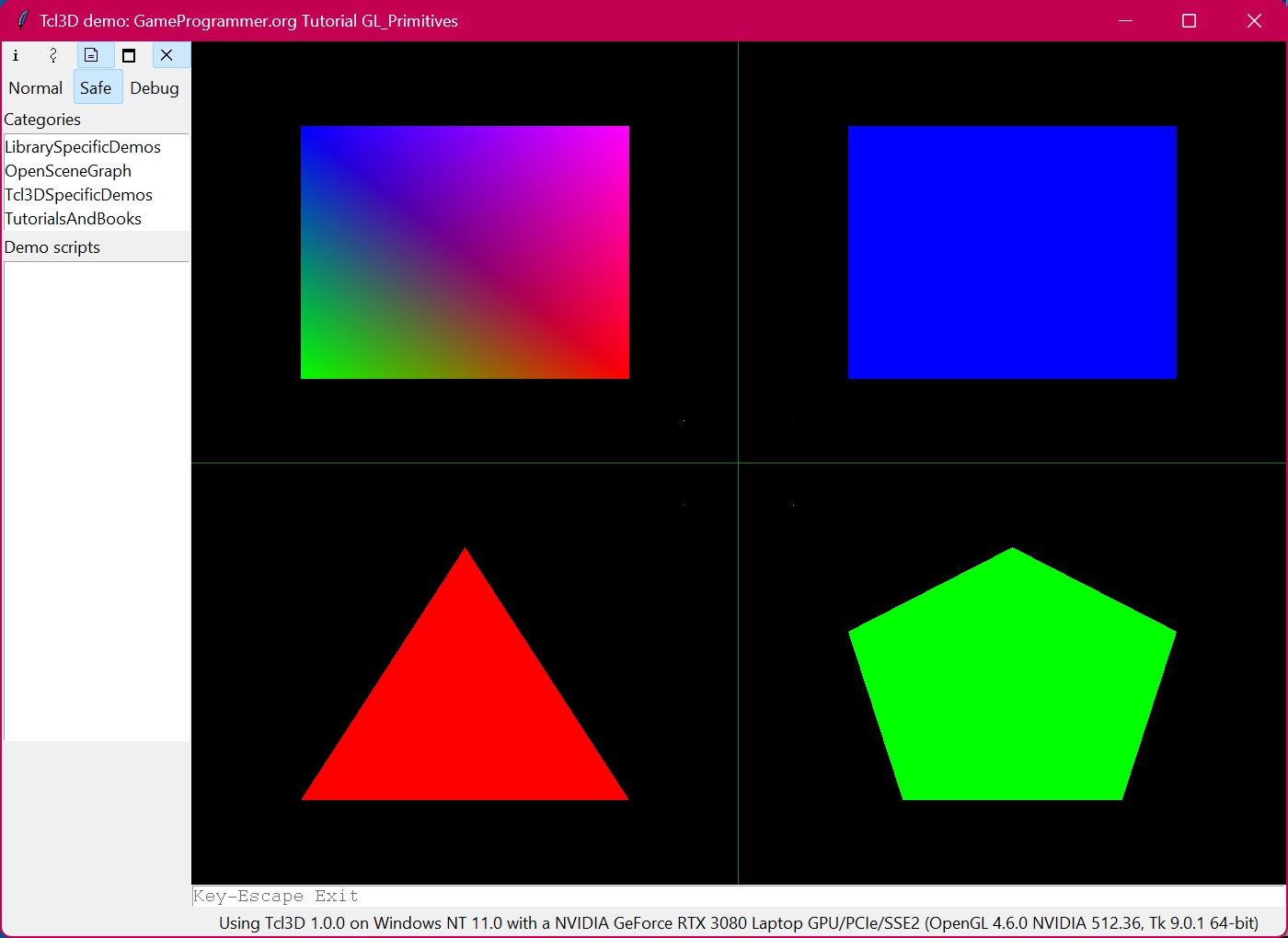 |
# GL_Primitives.tcl
#
# Tutorial from www.GameProgrammer.org
# OpenGL Primitives.
#
# Original code Copyright 2004 by Vahid Kazemi
#
# Modified for Tcl3D by Paul Obermeier 2006/09/11
# See www.tcl3d.org for the Tcl3D extension.
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Window size.
set winWidth 640
set winHeight 480
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
ExitProg
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
# The Togl callback function called when window is resized.
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
}
# The Togl callback function called when window is created.
proc CreateCallback { toglwin } {
;
}
# The Togl callback function for rendering a frame.
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
# Points
glBegin GL_POINTS
glColor3f 1 0 0 ; glVertex2f -0.1 -0.1 ; # red point
glColor3f 0 1 0 ; glVertex2f 0.1 -0.1 ; # green point
glColor3f 0 0 1 ; glVertex2f 0.1 0.1 ; # blue point
glColor3f 1 1 1 ; glVertex2f -0.1 0.1 ; # white point
glEnd
# Lines
glColor3f 0.1 0.5 0.1
glBegin GL_LINES
# Horziontal :
glVertex2f -1 0
glVertex2f 1 0
# Vertical :
glVertex2f 0 -1
glVertex2f 0 1
glEnd
# Red Triangle
glColor3f 1 0 0
glBegin GL_TRIANGLES
glVertex2f -0.5 -0.2
glVertex2f -0.8 -0.8
glVertex2f -0.2 -0.8
glEnd
# Blue Quad
glColor3f 0 0 1
glBegin GL_TRIANGLE_STRIP
glVertex2f 0.8 0.2
glVertex2f 0.2 0.2
glVertex2f 0.8 0.8
glVertex2f 0.2 0.8
glEnd
# Colorful Quad
glBegin GL_QUADS
glColor3f 1 0 0 ; glVertex2f -0.2 0.2
glColor3f 0 1 0 ; glVertex2f -0.8 0.2
glColor3f 0 0 1 ; glVertex2f -0.8 0.8
glColor3f 1 0 1 ; glVertex2f -0.2 0.8
glEnd
# Five Sided Polygon
glColor3f 0 1 0
glBegin GL_POLYGON
glVertex2f 0.5 -0.2
glVertex2f 0.8 -0.4
glVertex2f 0.7 -0.8
glVertex2f 0.3 -0.8
glVertex2f 0.2 -0.4
glEnd
glFlush
$toglwin swapbuffers
}
# Put all exit related code here.
proc ExitProg {} {
exit
}
# Create the OpenGL window and some Tk helper widgets.
proc CreateWindow {} {
frame .fr
pack .fr -expand 1 -fill both
# Create Our OpenGL Window
togl .fr.toglwin -width $::winWidth -height $::winHeight \
-double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 1
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: GameProgrammer.org Tutorial GL_Primitives"
# Watch For ESC Key And Quit Messages
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
}
CreateWindow
PrintInfo [tcl3dOglGetInfoString]
|
