Demo 66 of 68 in category RedBook
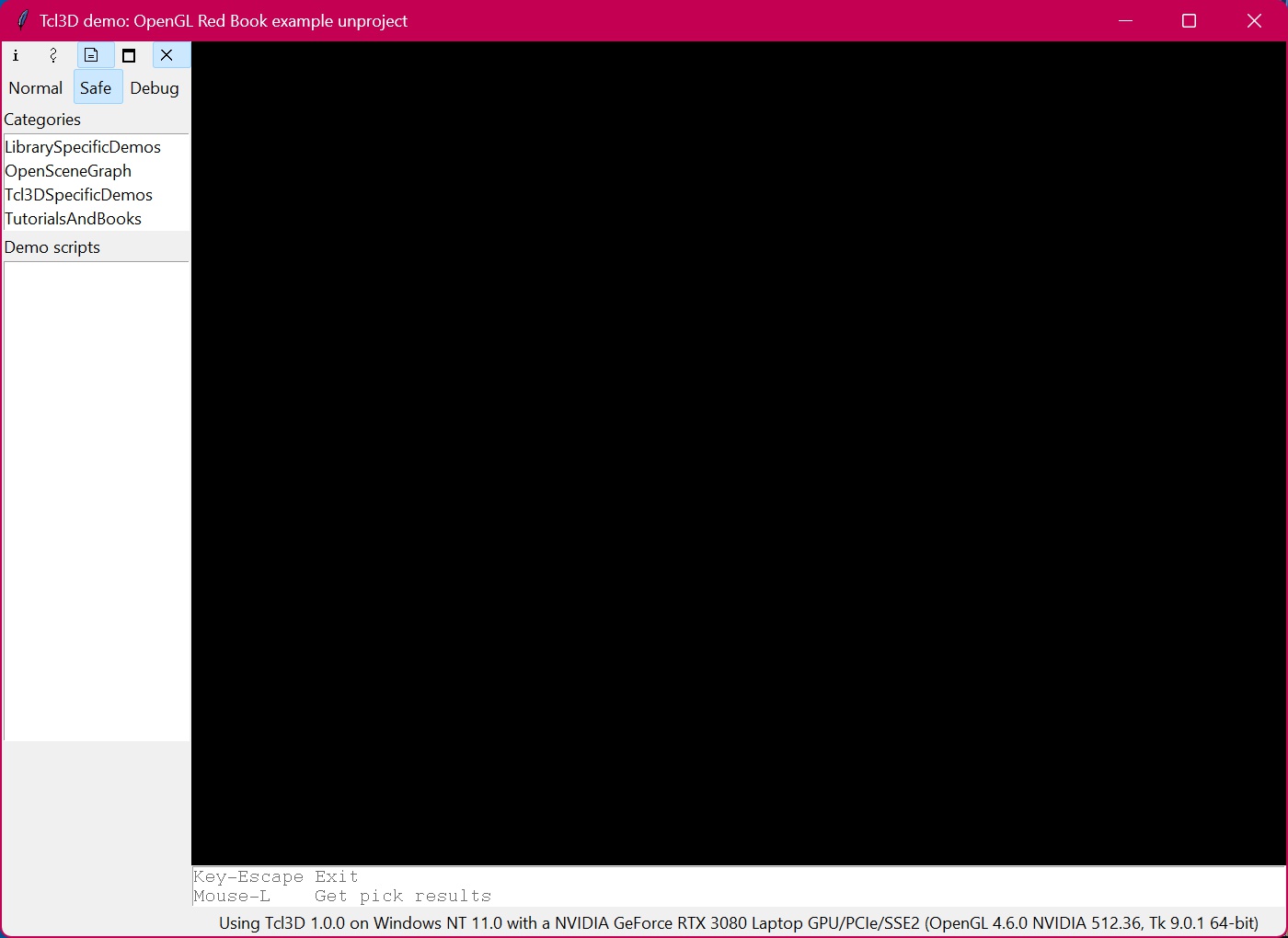 |
# unproject.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2025, Paul Obermeier.
# See file LICENSE for complete license information.
#
# When the left mouse button is pressed, this program
# reads the mouse position and determines two 3D points
# from which it was transformed. Very little is displayed.
package require tcl3d
tcl3dConsoleCreate .tcl3dOutputConsole "# " "Unproject Output"
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# tcl3dVectors used for the gluUnprojectVec function.
set gViewport [tcl3dVector GLint 4]
set gMvMatrix [tcl3dVector GLdouble 16]
set gProjMatrix [tcl3dVector GLdouble 16]
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
}
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 [expr double($w)/double($h)] 1.0 100.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
proc MouseDown { x y } {
global gViewport gMvMatrix gProjMatrix
glGetIntegerv GL_VIEWPORT $gViewport
glGetDoublev GL_MODELVIEW_MATRIX $gMvMatrix
glGetDoublev GL_PROJECTION_MATRIX $gProjMatrix
# Note: viewport[3] is height of window in pixels
set realy [expr [$gViewport get 3] - $y - 1]
puts "Coordinates at cursor are ($x, $realy)"
set winList [tcl3dOglUnProject $x $realy 0.0 $gMvMatrix $gProjMatrix $gViewport]
puts [format "World coords at z=0.0 are (%f, %f, %f)" \
[lindex $winList 1] [lindex $winList 2] [lindex $winList 3]]
set winList [tcl3dOglUnProject $x $realy 1.0 $gMvMatrix $gProjMatrix $gViewport]
puts [format "World coords at z=1.0 are (%f, %f, %f)" \
[lindex $winList 1] [lindex $winList 2] [lindex $winList 3]]
}
proc Cleanup {} {
global gViewport gMvMatrix gProjMatrix
$gViewport delete
$gMvMatrix delete
$gProjMatrix delete
uplevel #0 unset gViewport
uplevel #0 unset gMvMatrix
uplevel #0 unset gProjMatrix
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 2
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example unproject"
bind . <Key-Escape> "exit"
bind .fr.toglwin <1> "MouseDown %x %y"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Mouse-L Get pick results"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
