Demo 64 of 68 in category RedBook
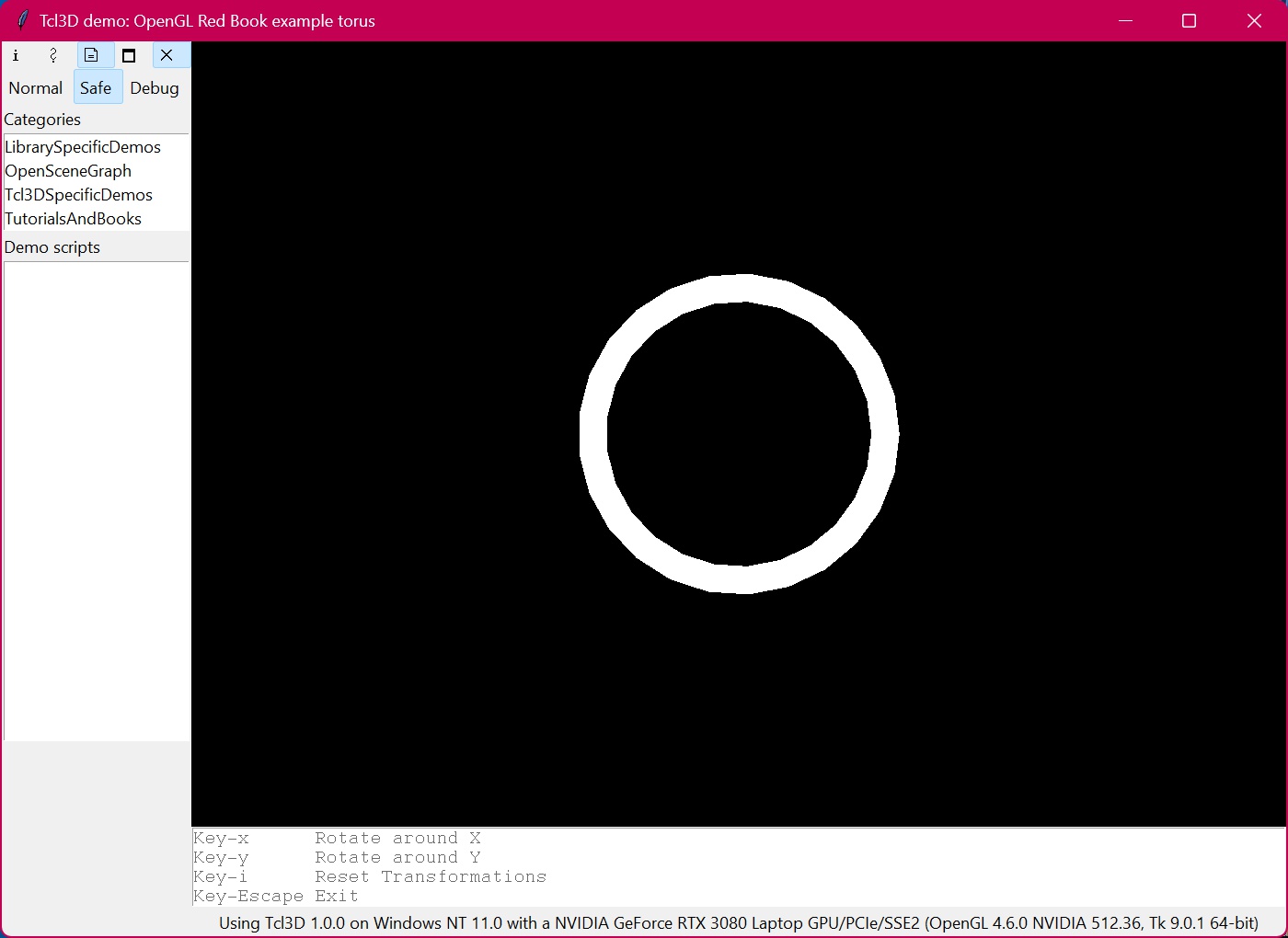 |
# torus.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program demonstrates the creation of a display list.
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
# Draw a torus
proc torus { numc numt } {
set PI 3.14159265358979323846
set twopi [expr 2 * $PI]
for { set i 0 } { $i < $numc } { incr i } {
glBegin GL_QUAD_STRIP
for {set j 0 } { $j <= $numt } { incr j } {
for { set k 1 } { $k >= 0 } { incr k -1 } {
set s [expr ($i + $k) % $numc + 0.5]
set t [expr $j % $numt]
set x [expr (1 + 0.1*cos($s*$twopi/$numc))*cos($t*$twopi/$numt)]
set y [expr (1 + 0.1*cos($s*$twopi/$numc))*sin($t*$twopi/$numt)]
set z [expr 0.1 * sin($s * $twopi / $numc)]
glVertex3f $x $y $z
}
}
glEnd
}
}
# Create display list with Torus and initialize state
proc CreateCallback { toglwin } {
global theTorus
set theTorus [glGenLists 1]
glNewList $theTorus GL_COMPILE
torus 8 25
glEndList
glShadeModel GL_FLAT
glClearColor 0.0 0.0 0.0 0.0
}
# Clear window and draw torus
proc DisplayCallback { toglwin } {
global theTorus
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glColor3f 1.0 1.0 1.0
glCallList $theTorus
glFlush
$toglwin swapbuffers
}
# Handle window resize
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 30 [expr double($w)/double($h)] 1.0 100.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
gluLookAt 0 0 10 0 0 0 0 1 0
}
proc RotX {} {
glRotatef 30.0 1.0 0.0 0.0
.fr.toglwin postredisplay
}
proc RotY {} {
glRotatef 30.0 0.0 1.0 0.0
.fr.toglwin postredisplay
}
proc ResetTfm {} {
glLoadIdentity
gluLookAt 0 0 10 0 0 0 0 1 0
.fr.toglwin postredisplay
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 400 -height 400 -double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 4
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example torus"
bind . <Key-x> "RotX"
bind . <Key-y> "RotY"
bind . <Key-i> "ResetTfm"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-x Rotate around X"
.fr.usage insert end "Key-y Rotate around Y"
.fr.usage insert end "Key-i Reset Transformations"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
