Demo 49 of 68 in category RedBook
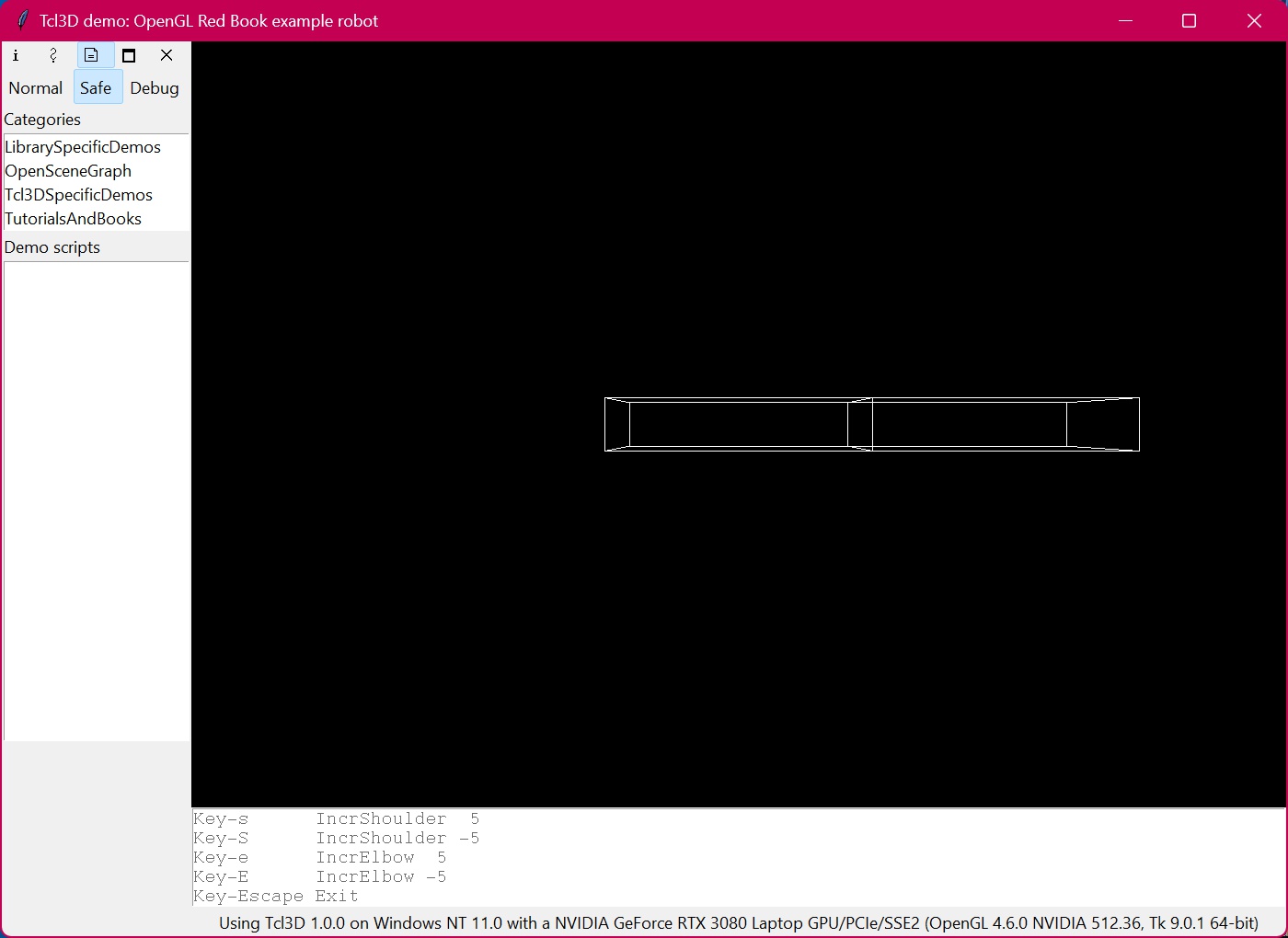 |
# robot.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program shows how to composite modeling transformations
# to draw translated and rotated hierarchical models.
# Interaction: pressing the s and e keys (shoulder and elbow)
# alters the rotation of the robot arm.
package require tcl3d
set shoulder 0
set elbow 0
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
glClearColor 0.0 0.0 0.0 0.0
glShadeModel GL_FLAT
}
proc DisplayCallback { toglwin } {
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glPushMatrix
glTranslatef -1.0 0.0 0.0
glRotatef $::shoulder 0.0 0.0 1.0
glTranslatef 1.0 0.0 0.0
glPushMatrix
glScalef 2.0 0.4 1.0
glutWireCube 1.0
glPopMatrix
glTranslatef 1.0 0.0 0.0
glRotatef $::elbow 0.0 0.0 1.0
glTranslatef 1.0 0.0 0.0
glPushMatrix
glScalef 2.0 0.4 1.0
glutWireCube 1.0
glPopMatrix
glPopMatrix
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 65.0 [expr double ($w) / double ($h)] 1.0 20.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
glTranslatef 0.0 0.0 -5.0
}
proc IncrShoulder { val } {
set ::shoulder [expr ($::shoulder + $val) % 360]
.fr.toglwin postredisplay
}
proc IncrElbow { val } {
set ::elbow [expr ($::elbow + $val) % 360]
.fr.toglwin postredisplay
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 5
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example robot"
bind . <Key-s> "IncrShoulder 5"
bind . <Key-S> "IncrShoulder -5"
bind . <Key-e> "IncrElbow 5"
bind . <Key-E> "IncrElbow -5"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-s IncrShoulder 5"
.fr.usage insert end "Key-S IncrShoulder -5"
.fr.usage insert end "Key-e IncrElbow 5"
.fr.usage insert end "Key-E IncrElbow -5"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
