Demo 45 of 68 in category RedBook
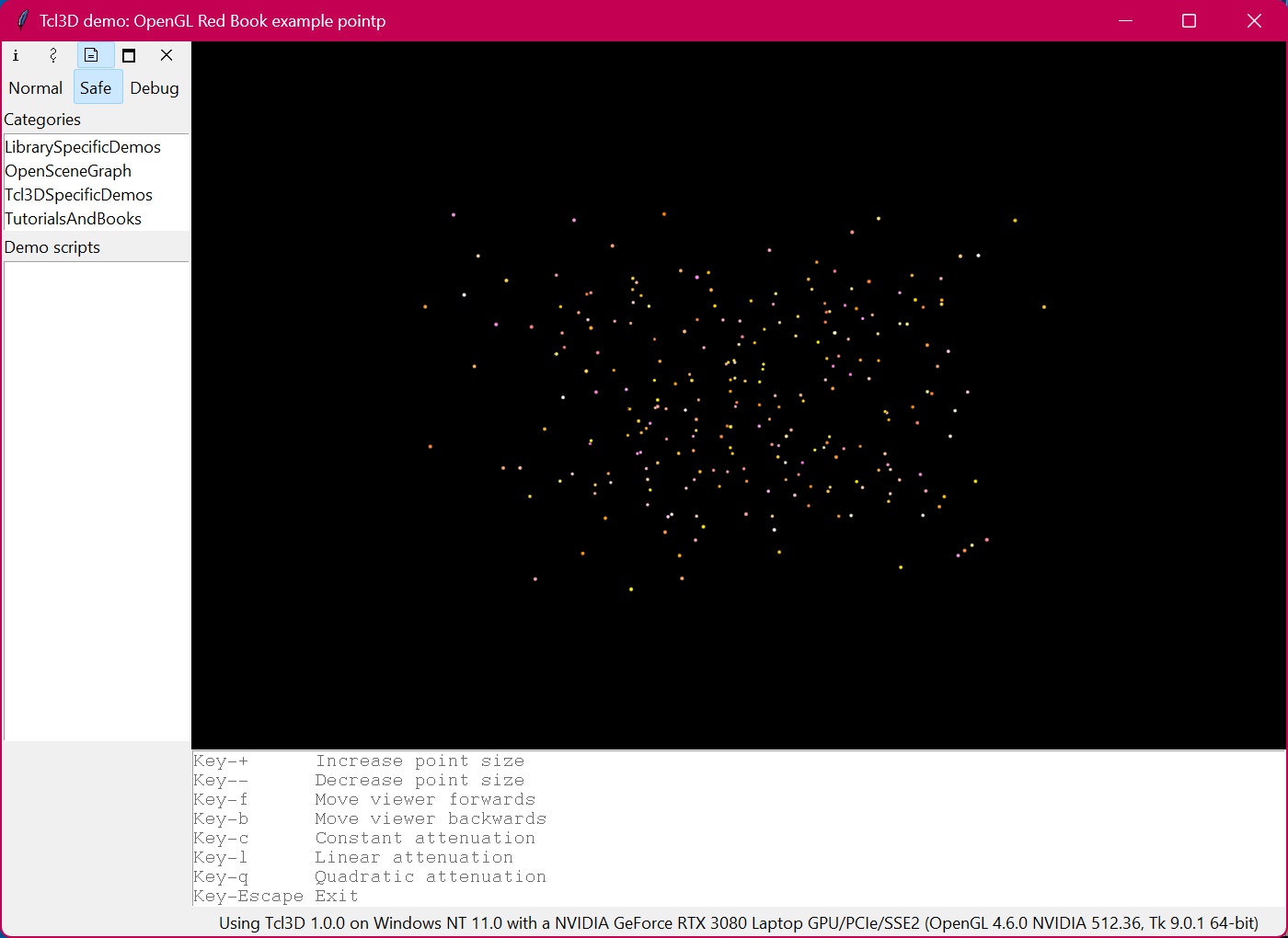 |
# pointp.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program demonstrates point parameters and their effect
# on point primitives.
# 250 points are randomly generated within a 10 by 10 by 40
# region, centered at the origin. In some modes (including the
# default), points that are closer to the viewer will appear larger.
#
# Pressing the 'l', 'q', and 'c' keys switch the point
# parameters attenuation mode to linear, quadratic, or constant,
# respectively.
# Pressing the 'f' and 'b' keys move the viewer forward
# and backwards. In either linear or quadratic attenuation
# mode, the distance from the viewer to the point will change
# the size of the point primitive.
# Pressing the '+' and '-' keys will change the current point
# size. In this program, the point size is bounded, so it
# will not get less than 2.0, nor greater than GL_POINT_SIZE_MAX.
package require tcl3d
set psize 7.0
set constant {1.0 0.0 0.0}
set linear {0.0 0.12 0.0}
set quadratic {0.0 0.0 0.01}
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
expr srand (12345)
glNewList 1 GL_COMPILE
glBegin GL_POINTS
for { set i 0 } { $i < 250 } { incr i } {
glColor3f 1.0 [expr rand() * 0.5 + 0.5] [expr rand()]
# randomly generated vertices:
# -5 < x < 5; -5 < y < 5; -5 < z < -45 */
glVertex3f [expr rand() * 10.0 - 5.0] \
[expr rand() * 10.0 - 5.0] \
[expr rand() * 40.0 - 45.0]
}
glEnd
glEndList
glEnable GL_DEPTH_TEST
glEnable GL_POINT_SMOOTH
glEnable GL_BLEND
glBlendFunc GL_SRC_ALPHA GL_ONE_MINUS_SRC_ALPHA
glPointSize $::psize
set ::pmax [tcl3dOglGetFloatState GL_POINT_SIZE_MAX]
glPointParameterfv GL_POINT_DISTANCE_ATTENUATION $::linear
glPointParameterf GL_POINT_FADE_THRESHOLD_SIZE 2.0
}
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glCallList 1
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 35.0 1.0 0.25 200.0
glMatrixMode GL_MODELVIEW
glTranslatef 0.0 0.0 -10.0
}
proc setAttenuationMode { toglwin mode } {
glPointParameterfv GL_POINT_DISTANCE_ATTENUATION $mode
$toglwin postredisplay
}
proc moveViewer { toglwin dz } {
glMatrixMode GL_MODELVIEW
glTranslatef 0.0 0.0 $dz
$toglwin postredisplay
}
proc setPointSize { toglwin ds } {
if { $ds < 0.0 } {
if { $::psize >= 2.0 } {
set ::psize [expr $::psize + $ds]
}
} else {
if { $::psize < ($::pmax + 1.0) } {
set ::psize [expr $::psize + $ds]
}
}
glPointSize $::psize
$toglwin postredisplay
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 8
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example pointp"
bind . <Key-plus> "setPointSize .fr.toglwin 1.0"
bind . <Key-minus> "setPointSize .fr.toglwin -1.0"
bind . <Key-f> "moveViewer .fr.toglwin 0.5"
bind . <Key-b> "moveViewer .fr.toglwin -0.5"
bind . <Key-c> "setAttenuationMode .fr.toglwin [list $constant]"
bind . <Key-l> "setAttenuationMode .fr.toglwin [list $linear]"
bind . <Key-q> "setAttenuationMode .fr.toglwin [list $quadratic]"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-+ Increase point size"
.fr.usage insert end "Key-- Decrease point size"
.fr.usage insert end "Key-f Move viewer forwards"
.fr.usage insert end "Key-b Move viewer backwards"
.fr.usage insert end "Key-c Constant attenuation"
.fr.usage insert end "Key-l Linear attenuation"
.fr.usage insert end "Key-q Quadratic attenuation"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
if { ! [tcl3dOglHaveVersion 1 4] } {
tk_messageBox -icon warning -type ok -title "Invalid OpenGL version" \
-message [format "Feature needs OpenGL >= 1.4. Only have %s" \
[glGetString GL_VERSION]]
}
|
