Demo 29 of 68 in category RedBook
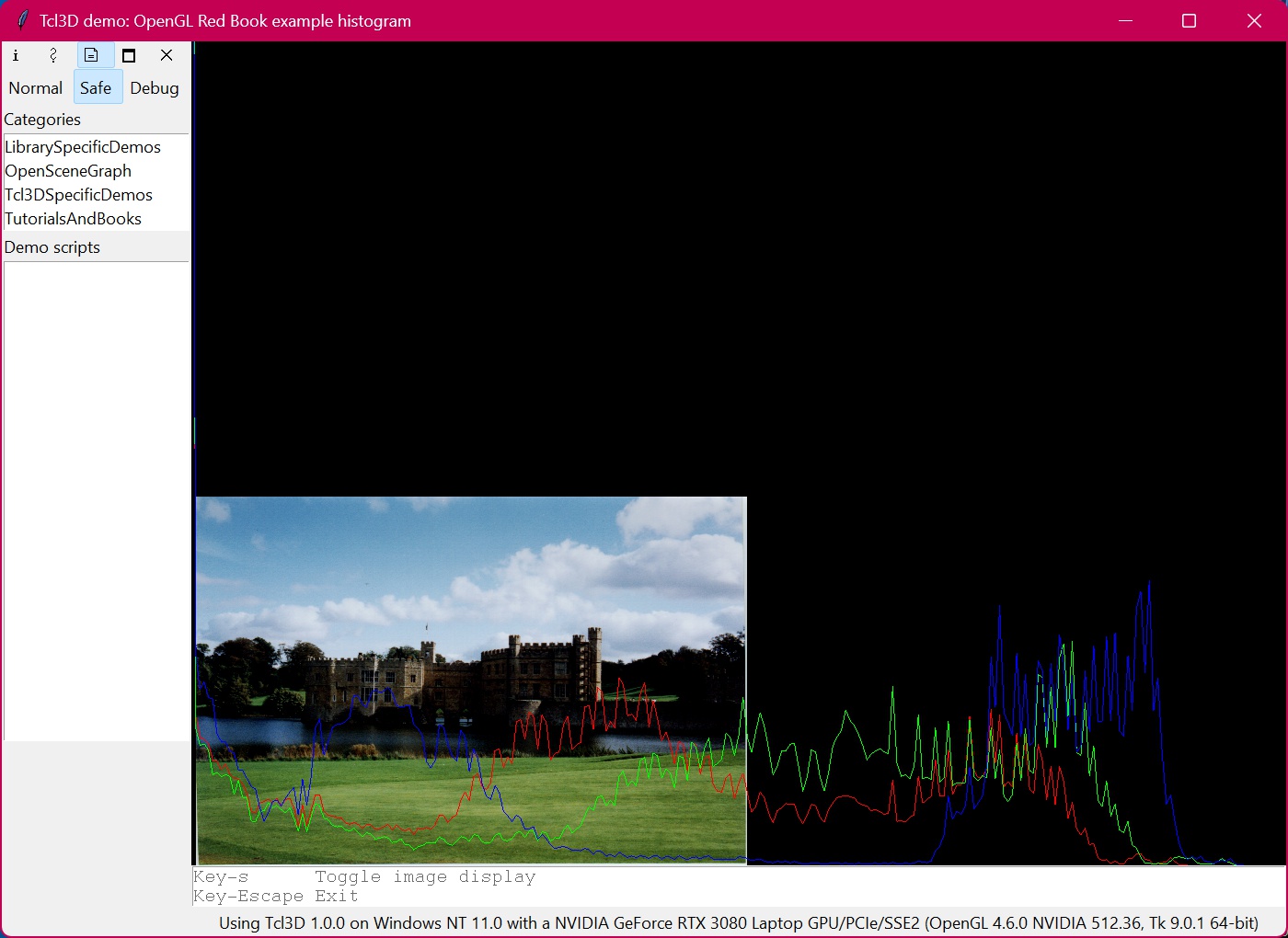 |
# histogram.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2022, Paul Obermeier.
# See file LICENSE for complete license information.
#
# Compute the histogram of the image. This program illustrates the
# use of the glHistogram() function.
package require tcl3d
set HISTOGRAM_SIZE 256 ; # Must be a power of 2
set sink 0
set gHaveNeededExtensions true
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
if { ! [tcl3dOglHaveExtension $toglwin "GL_ARB_imaging"] } {
set ::gHaveNeededExtensions false
tk_messageBox -icon error -type ok -title "Missing OpenGL extension" \
-message "Demo needs the GL_ARB_imaging extension."
proc ::Cleanup {} {}
exit 1
return
}
glPixelStorei GL_UNPACK_ALIGNMENT 1
glClearColor 0.0 0.0 0.0 0.0
glHistogram GL_HISTOGRAM $::HISTOGRAM_SIZE GL_RGB GL_FALSE
glEnable GL_HISTOGRAM
}
proc DisplayCallback { toglwin } {
if { $::gHaveNeededExtensions == false } {
return
}
set values [tcl3dVector GLushort [expr $::HISTOGRAM_SIZE*3]]
glClear GL_COLOR_BUFFER_BIT
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glRasterPos2i 1 1
glDrawPixels $::width $::height GL_RGB GL_UNSIGNED_BYTE $::pixels
glGetHistogram GL_HISTOGRAM GL_TRUE GL_RGB GL_UNSIGNED_SHORT $values
# Plot histogram
glBegin GL_LINE_STRIP
glColor3f 1.0 0.0 0.0
for { set i 0 } { $i < $::HISTOGRAM_SIZE } { incr i } {
glVertex2s $i [$values get [expr $i*3 + 0]]
}
glEnd
glBegin GL_LINE_STRIP
glColor3f 0.0 1.0 0.0
for { set i 0 } { $i < $::HISTOGRAM_SIZE } { incr i } {
glVertex2s $i [$values get [expr $i*3 + 1]]
}
glEnd
glBegin GL_LINE_STRIP
glColor3f 0.0 0.0 1.0
for { set i 0 } { $i < $::HISTOGRAM_SIZE } { incr i } {
glVertex2s $i [$values get [expr $i*3 + 2]]
}
glEnd
glFlush
$values delete
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
if { $::gHaveNeededExtensions == false } {
return
}
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
glOrtho 0 256 0 10000 -1.0 1.0
glMatrixMode GL_MODELVIEW
}
proc toggleSink { toglwin } {
set ::sink [expr 1 - $::sink]
glHistogram GL_HISTOGRAM $::HISTOGRAM_SIZE GL_RGB $::sink
$toglwin postredisplay
}
set widthVec [tcl3dVector GLsizei 1]
set heightVec [tcl3dVector GLsizei 1]
set imgName [file join [file dirname [info script]] "Data" "leeds.bin"]
set pixels [tcl3dReadRedBookImage [tcl3dGetExtFile $imgName] $widthVec $heightVec]
set width [$widthVec get 0]
set height [$heightVec get 0]
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width $width -height $height \
-double true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 2
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example histogram"
bind . <Key-s> "toggleSink .fr.toglwin"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-s Toggle image display"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
