Demo 7 of 68 in category RedBook
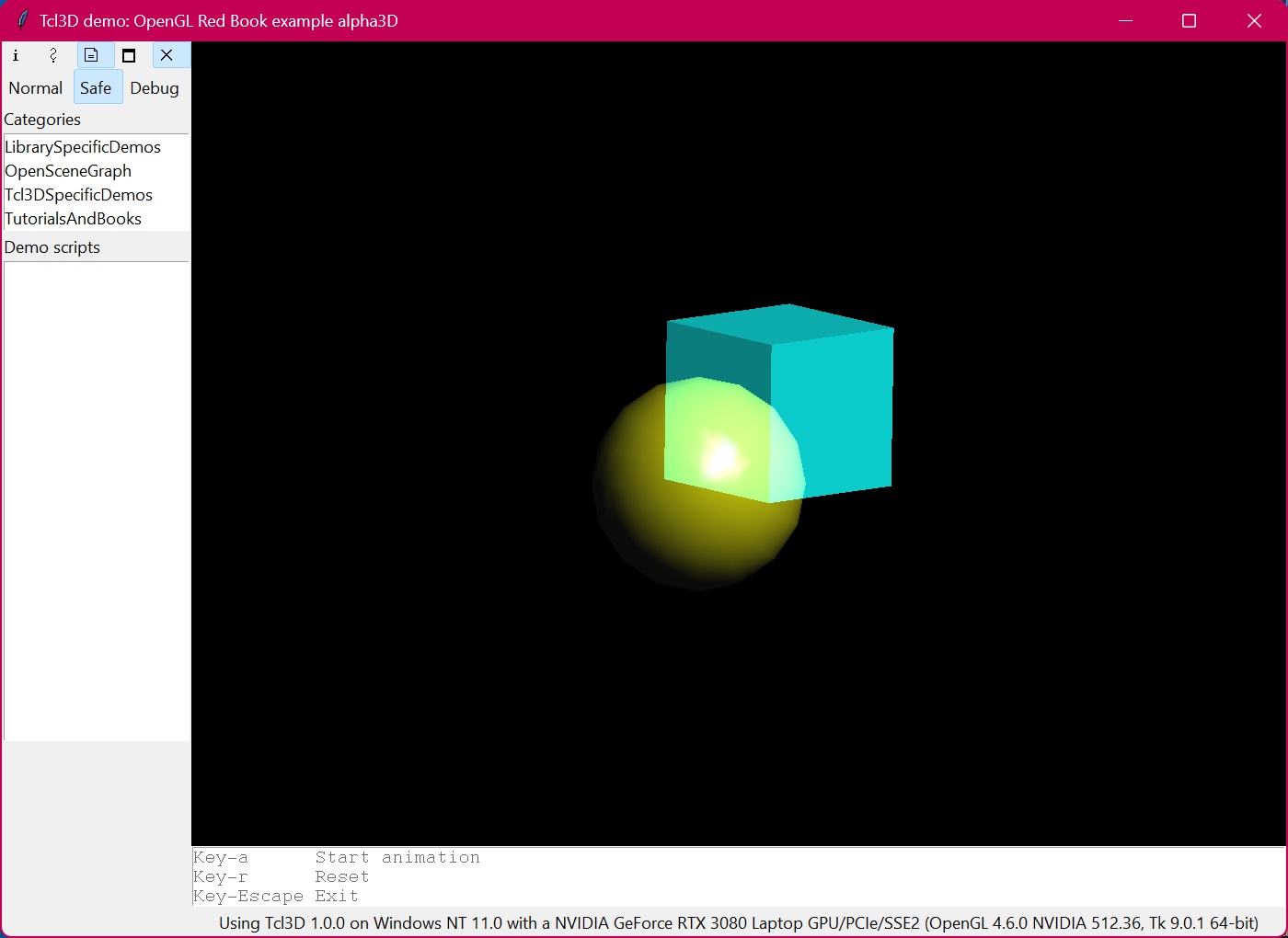 |
# alpha3D.tcl
#
# An example of the OpenGL red book modified to work with Tcl3D.
# The original C sources are Copyright (c) 1993-2003, Silicon Graphics, Inc.
# The Tcl3D sources are Copyright (c) 2005-2025, Paul Obermeier.
# See file LICENSE for complete license information.
#
# This program demonstrates how to intermix opaque and
# alpha blended polygons in the same scene, by using
# glDepthMask. Press the 'a' key to animate moving the
# transparent object through the opaque object. Press
# the 'r' key to reset the scene.
package require tcl3d
set MAXZ 8.0
set MINZ -8.0
set ZINC 0.4
set solidZ $MAXZ
set transparentZ $MINZ
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc CreateCallback { toglwin } {
global sphereList cubeList
set mat_specular { 1.0 1.0 1.0 0.15 }
set mat_shininess { 100.0 }
set position { 0.5 0.5 1.0 0.0 }
glMaterialfv GL_FRONT GL_SPECULAR $mat_specular
glMaterialfv GL_FRONT GL_SHININESS $mat_shininess
glLightfv GL_LIGHT0 GL_POSITION $position
glEnable GL_LIGHTING
glEnable GL_LIGHT0
glEnable GL_DEPTH_TEST
set sphereList [glGenLists 1]
glNewList $sphereList GL_COMPILE
glutSolidSphere 0.4 16 16
glEndList
set cubeList [glGenLists 1]
glNewList $cubeList GL_COMPILE
glutSolidCube 0.6
glEndList
}
proc DisplayCallback { toglwin } {
global sphereList cubeList
set mat_solid { 0.75 0.75 0.0 1.0 }
set mat_zero { 0.0 0.0 0.0 1.0 }
set mat_transparent { 0.0 0.8 0.8 0.6 }
set mat_emission { 0.0 0.3 0.3 0.6 }
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glPushMatrix
glTranslatef -0.15 -0.15 $::solidZ
glMaterialfv GL_FRONT GL_EMISSION $mat_zero
glMaterialfv GL_FRONT GL_DIFFUSE $mat_solid
glCallList $sphereList
glPopMatrix
glPushMatrix
glTranslatef 0.15 0.15 $::transparentZ
glRotatef 15.0 1.0 1.0 0.0
glRotatef 30.0 0.0 1.0 0.0
glMaterialfv GL_FRONT GL_EMISSION $mat_emission
glMaterialfv GL_FRONT GL_DIFFUSE $mat_transparent
glEnable GL_BLEND
glDepthMask GL_FALSE
glBlendFunc GL_SRC_ALPHA GL_ONE
glCallList $cubeList
glDepthMask GL_TRUE
glDisable GL_BLEND
glPopMatrix
glFlush
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
if { $w <= $h } {
glOrtho -1.5 1.5 [expr -1.5*double($h)/double($w)] \
[expr 1.5*double($h)/double($w)] -10.0 10.0
} else {
glOrtho [expr -1.5*double($w)/double($h)] \
[expr 1.5*double($w)/double($h)] -1.5 1.5 -10.0 10.0
}
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
proc Animate {} {
if { $::solidZ <= $::MINZ || $::transparentZ >= $::MAXZ } {
;
} else {
set ::solidZ [expr $::solidZ - $::ZINC]
set ::transparentZ [expr $::transparentZ + $::ZINC]
.fr.toglwin postredisplay
tcl3dAfterIdle Animate
}
}
proc Reset {} {
set ::solidZ $::MAXZ
set ::transparentZ $::MINZ
.fr.toglwin postredisplay
}
proc StartAnimation {} {
set ::solidZ $::MAXZ
set ::transparentZ $::MINZ
Animate
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width 500 -height 500 -double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::listFont -height 3
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: OpenGL Red Book example alpha3D"
bind . <Key-a> "StartAnimation"
bind . <Key-r> "Reset"
bind . <Key-Escape> "exit"
.fr.usage insert end "Key-a Start animation"
.fr.usage insert end "Key-r Reset"
.fr.usage insert end "Key-Escape Exit"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
