Demo ogl_alpha_blending_framebuffer
Demo 1 of 19 in category CodeSampler
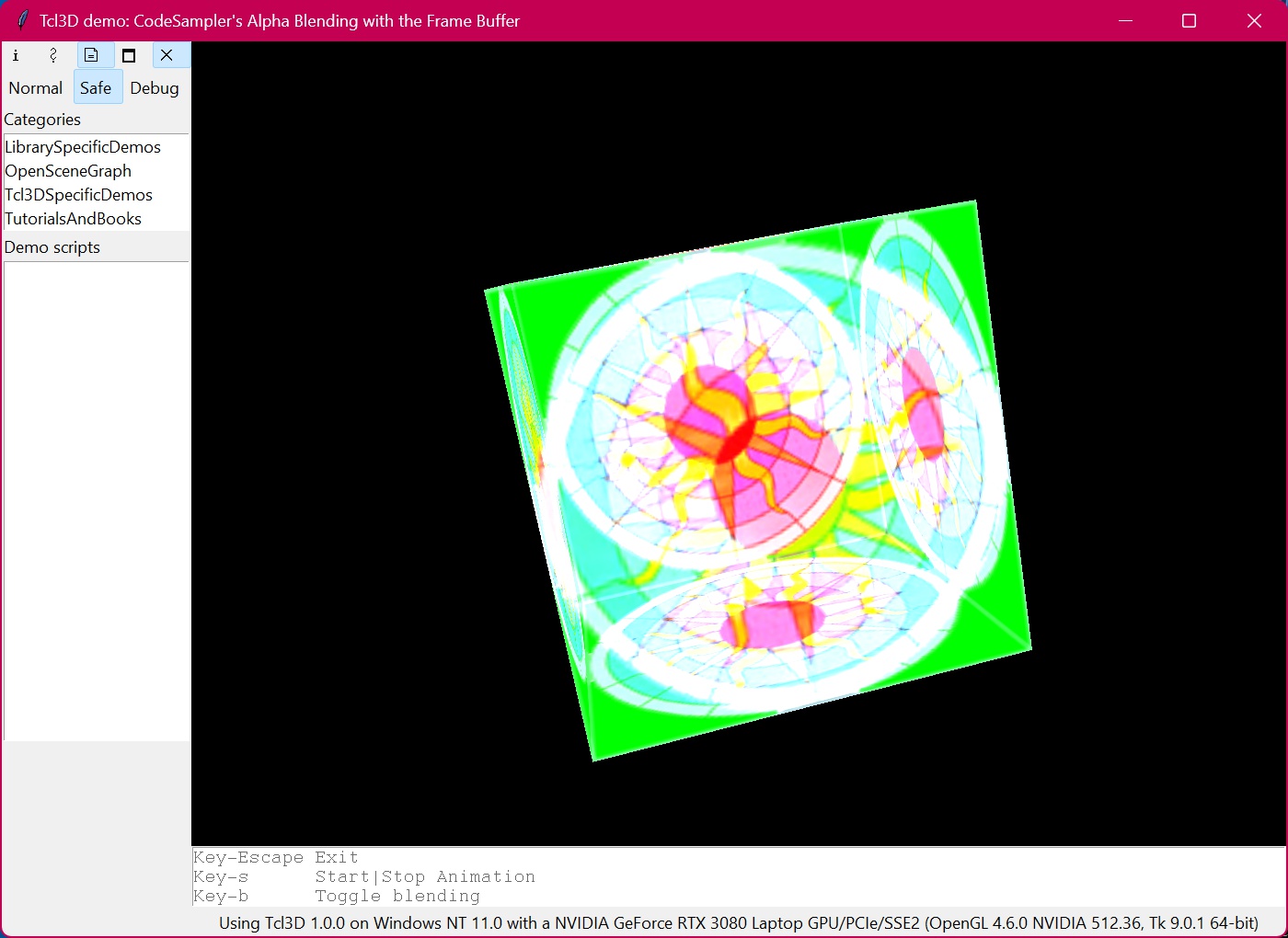 |
#-----------------------------------------------------------------------------
# Name: ogl_alpha_blending_framebuffer.cpp
# Author: Kevin Harris (kevin@codesampler.com)
# Last Modified: 03/25/05
# Description: This sample demonstrates how to perform alpha-blending in
# the frame-buffer. The sample renders a textured cube which
# is alpha-blended into the frame-buffer in such a way as to
# create a translucent effect.
#
# Control Keys: b - Toggle blending
#-----------------------------------------------------------------------------
#
# Original C++ code by Kevin Harris (kevin@codesampler.com)
# See www.codesampler.com for the original files
# OpenGL samples page 4: Alpha Blending in the Frame buffer
# http://www.codesampler.com/oglsrc/oglsrc_4.htm#ogl_alpha_blending_framebuffer
#
# Modified for Tcl3D by Paul Obermeier 2008/05/01
# See www.tcl3d.org for the Tcl3D extension.
package require Tk
package require Img
package require tcl3d
# Font to be used in the Tk listbox.
set g_listFont {-family {Courier} -size 10}
set g_WinWidth 640
set g_WinHeight 480
set g_StopWatch [tcl3dNewSwatch]
set g_fLastTime 0.0
set g_bBlending true
set g_bAnimStarted false
set ::g_fXrot 0.0
set ::g_fYrot 0.0
set ::g_fZrot 0.0
# Array of texture coordinates and vertices (GL_T2F_V3F)
# for glInterleavedArrays call.
set g_cubeVertices [tcl3dVectorFromArgs GLfloat \
0.0 0.0 -1.0 -1.0 1.0 \
1.0 0.0 1.0 -1.0 1.0 \
1.0 1.0 1.0 1.0 1.0 \
0.0 1.0 -1.0 1.0 1.0 \
\
1.0 0.0 -1.0 -1.0 -1.0 \
1.0 1.0 -1.0 1.0 -1.0 \
0.0 1.0 1.0 1.0 -1.0 \
0.0 0.0 1.0 -1.0 -1.0 \
\
0.0 1.0 -1.0 1.0 -1.0 \
0.0 0.0 -1.0 1.0 1.0 \
1.0 0.0 1.0 1.0 1.0 \
1.0 1.0 1.0 1.0 -1.0 \
\
1.0 1.0 -1.0 -1.0 -1.0 \
0.0 1.0 1.0 -1.0 -1.0 \
0.0 0.0 1.0 -1.0 1.0 \
1.0 0.0 -1.0 -1.0 1.0 \
\
1.0 0.0 1.0 -1.0 -1.0 \
1.0 1.0 1.0 1.0 -1.0 \
0.0 1.0 1.0 1.0 1.0 \
0.0 0.0 1.0 -1.0 1.0 \
\
0.0 0.0 -1.0 -1.0 -1.0 \
1.0 0.0 -1.0 -1.0 1.0 \
1.0 1.0 -1.0 1.0 1.0 \
0.0 1.0 -1.0 1.0 -1.0 \
]
# Determine the directory of this script.
set g_scriptDir [file dirname [info script]]
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc ToggleBlending {} {
set ::g_bBlending [expr ! $::g_bBlending]
.fr.toglwin postredisplay
}
proc LoadTexture {} {
set texName [file join $::g_scriptDir "glass.bmp"]
set retVal [catch {set phImg [image create photo -file $texName]} err1]
if { $retVal != 0 } {
error "Error reading image $texName ($err1)"
} else {
set w [image width $phImg]
set h [image height $phImg]
set n [tcl3dPhotoChans $phImg]
set pTextureImage [tcl3dVectorFromPhoto $phImg]
image delete $phImg
}
set ::g_textureID [tcl3dVector GLuint 1]
glGenTextures 1 $::g_textureID
glBindTexture GL_TEXTURE_2D [$::g_textureID get 0]
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MIN_FILTER $::GL_LINEAR
glTexParameteri GL_TEXTURE_2D GL_TEXTURE_MAG_FILTER $::GL_LINEAR
if { $n == 3 } {
set type $::GL_RGB
} else {
set type $::GL_RGBA
}
glTexImage2D GL_TEXTURE_2D 0 $n $w $h 0 $type GL_UNSIGNED_BYTE $pTextureImage
$pTextureImage delete
}
proc CreateCallback { toglwin } {
LoadTexture
glClearColor 0.0 0.0 0.0 1.0
glEnable GL_TEXTURE_2D
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 [expr double($::g_WinWidth)/double($::g_WinHeight)] \
0.1 100.0
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 [expr double($w)/double($h)] 0.1 100.0
}
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
set ::g_fCurrentTime [tcl3dLookupSwatch $::g_StopWatch]
set ::g_fElapsedTime [expr {$::g_fCurrentTime - $::g_fLastTime}]
set ::g_fLastTime $::g_fCurrentTime
set ::g_fXrot [expr {$::g_fXrot + 10.1 * $::g_fElapsedTime}]
set ::g_fYrot [expr {$::g_fYrot + 10.2 * $::g_fElapsedTime}]
set ::g_fZrot [expr {$::g_fZrot + 10.3 * $::g_fElapsedTime}]
glMatrixMode GL_MODELVIEW
glLoadIdentity
glTranslatef 0.0 0.0 -5.0
glRotatef $::g_fXrot 1.0 0.0 0.0
glRotatef $::g_fYrot 0.0 1.0 0.0
glRotatef $::g_fZrot 0.0 0.0 1.0
if { $::g_bBlending } {
glDisable GL_DEPTH_TEST
# Set up blending...
glEnable GL_BLEND
glBlendFunc GL_SRC_ALPHA GL_ONE
glDisable GL_DEPTH_TEST
} else {
glDisable GL_BLEND
glEnable GL_DEPTH_TEST
}
glBindTexture GL_TEXTURE_2D [$::g_textureID get 0]
glInterleavedArrays GL_T2F_V3F 0 $::g_cubeVertices
glDrawArrays GL_QUADS 0 24
$toglwin swapbuffers
}
proc StartStopAnimation {} {
if { $::g_bAnimStarted == false } {
StartAnimation
} else {
StopAnimation
}
}
proc StartAnimation {} {
tcl3dStartSwatch $::g_StopWatch
.fr.toglwin postredisplay
set ::animId [tcl3dAfterIdle StartAnimation]
set ::g_bAnimStarted true
}
proc StopAnimation {} {
if { [info exists ::animId] } {
after cancel $::animId
unset ::animId
}
set ::g_bAnimStarted false
tcl3dStopSwatch $::g_StopWatch
}
proc Cleanup {} {
if { [info exists ::g_textureID] } {
glDeleteTextures 1 [$::g_textureID get 0]
$::g_textureID delete
}
$::g_cubeVertices delete
tcl3dDeleteSwatch $::g_StopWatch
foreach var [info globals g_*] {
uplevel #0 unset $var
}
}
proc ExitProg {} {
exit
}
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width $g_WinWidth -height $g_WinHeight \
-double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::g_listFont -height 3
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
set appTitle "Tcl3D demo: CodeSampler's Alpha Blending with the Frame Buffer"
wm title . $appTitle
# Watch For ESC Key And Quit Messages
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
bind . <Key-s> "StartStopAnimation"
bind . <Key-b> "ToggleBlending"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Key-s Start|Stop Animation"
.fr.usage insert end "Key-b Toggle blending"
.fr.usage configure -state disabled
PrintInfo [tcl3dOglGetInfoString]
|
