Demo 14 of 15 in category Tcl3DSpecificDemos
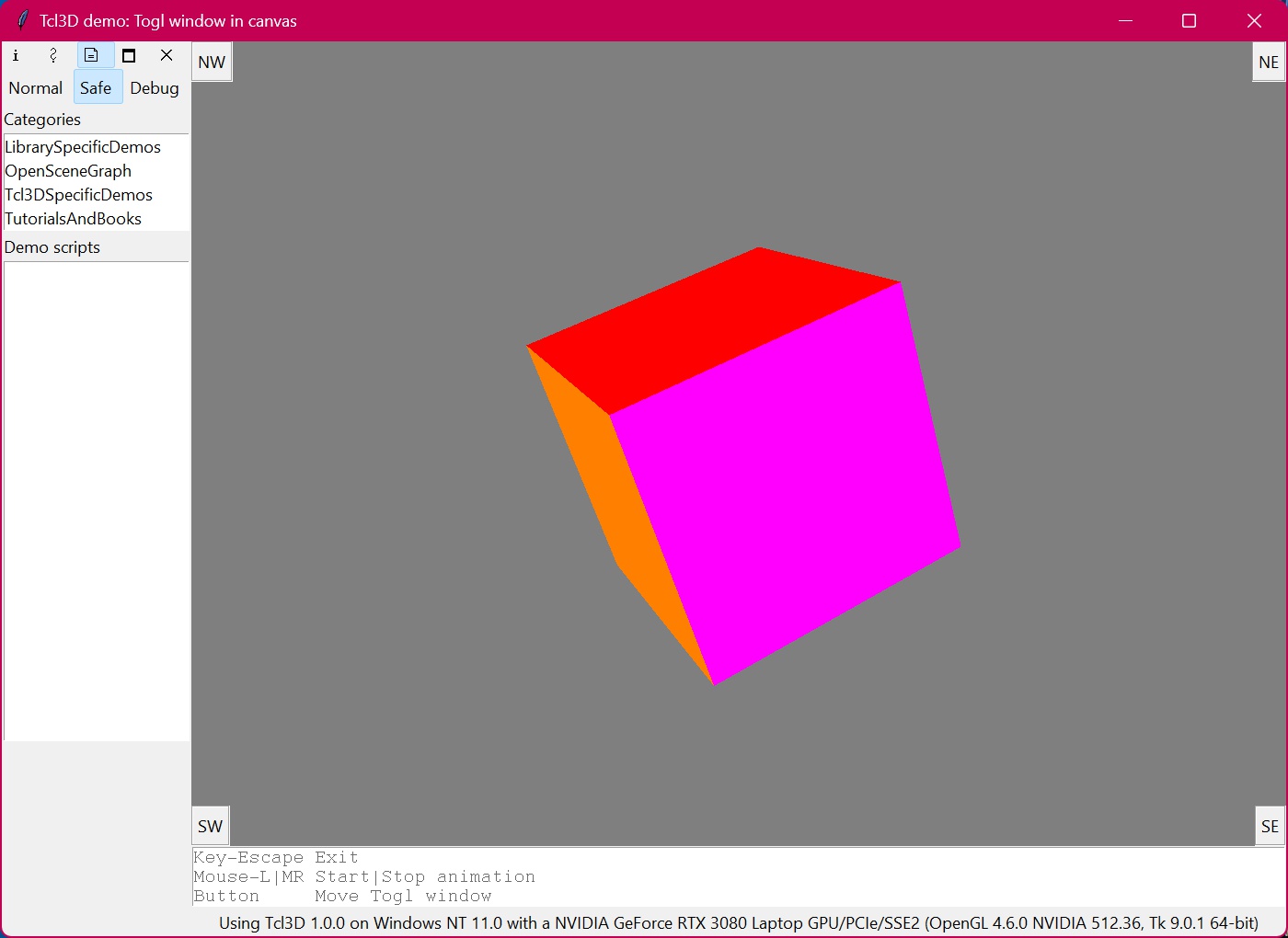 |
# toglInCanvas.tcl
#
# Tcl3D demo using a Togl window and some button widgets inserted into a canvas.
#
# Author: Paul Obermeier
# Date: 2006-12-08
package require Tk
package require tcl3d
# Font to be used in the Tk listbox.
set listFont {-family {Courier} -size 10}
# Rotation of the box
set g_RotAngle 0.0
# Offset of the Togl window.
set g_OffWidth 0
set g_OffHeight 0
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
exit
}
# Print info message into widget at the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc ShiftImg { dir } {
global g_MyCanv g_OffWidth g_OffHeight
switch $dir {
"SE" { set g_OffWidth [expr $g_OffWidth + 10]
set g_OffHeight [expr $g_OffHeight + 10]
}
"NE" { set g_OffWidth [expr $g_OffWidth + 10]
set g_OffHeight [expr $g_OffHeight - 10]
}
"SW" { set g_OffWidth [expr $g_OffWidth - 10]
set g_OffHeight [expr $g_OffHeight + 10]
}
"NW" { set g_OffWidth [expr $g_OffWidth - 10]
set g_OffHeight [expr $g_OffHeight - 10]
}
}
ReshapeCanvas $g_MyCanv
}
proc CreateCallback { toglwin } {
glClearColor 0.5 0.5 0.5 0.5
glShadeModel GL_SMOOTH
glClearDepth 1.0
glEnable GL_DEPTH_TEST
}
proc DisplayCallback { toglwin } {
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
glLoadIdentity
glTranslatef 0.0 0.0 -7.0
glRotatef $::g_RotAngle 1.0 1.0 1.0
glBegin GL_QUADS
glColor3f 0.0 1.0 0.0
glVertex3f 1.0 1.0 -1.0
glVertex3f -1.0 1.0 -1.0
glVertex3f -1.0 1.0 1.0
glVertex3f 1.0 1.0 1.0
glColor3f 1.0 0.5 0.0
glVertex3f 1.0 -1.0 1.0
glVertex3f -1.0 -1.0 1.0
glVertex3f -1.0 -1.0 -1.0
glVertex3f 1.0 -1.0 -1.0
glColor3f 1.0 0.0 0.0
glVertex3f 1.0 1.0 1.0
glVertex3f -1.0 1.0 1.0
glVertex3f -1.0 -1.0 1.0
glVertex3f 1.0 -1.0 1.0
glColor3f 1.0 1.0 0.0
glVertex3f 1.0 -1.0 -1.0
glVertex3f -1.0 -1.0 -1.0
glVertex3f -1.0 1.0 -1.0
glVertex3f 1.0 1.0 -1.0
glColor3f 0.0 0.0 1.0
glVertex3f -1.0 1.0 1.0
glVertex3f -1.0 1.0 -1.0
glVertex3f -1.0 -1.0 -1.0
glVertex3f -1.0 -1.0 1.0
glColor3f 1.0 0.0 1.0
glVertex3f 1.0 1.0 -1.0
glVertex3f 1.0 1.0 1.0
glVertex3f 1.0 -1.0 1.0
glVertex3f 1.0 -1.0 -1.0
glEnd
set ::g_RotAngle [expr $::g_RotAngle -0.5]
$toglwin swapbuffers
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 [expr double($w)/double($h)] 0.1 100.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
}
proc ReshapeCanvas { c } {
set w [winfo width $c]
set h [winfo height $c]
set cw [expr $w / 2]
set ch [expr $h / 2]
$c coords TOGLWIN [expr $cw + $::g_OffWidth] [expr $ch + $::g_OffHeight]
$c itemconfigure TOGLWIN -width $w -height $h
$c coords SE $w $h
$c coords SW 0 $h
$c coords NE $w 0
$c coords NW 0 0
$c coords TEXT1 $cw 20
$c coords TEXT2 $cw [expr $h -20]
}
proc Animate {} {
global g_MyCanv
$g_MyCanv.toglwin postredisplay
set ::animateId [tcl3dAfterIdle Animate]
}
proc StartAnimation {} {
if { ! [info exists ::animateId] } {
Animate
}
}
proc StopAnimation {} {
if { [info exists ::animateId] } {
after cancel $::animateId
unset ::animateId
}
}
proc Cleanup {} {
foreach var [info globals g_*] {
uplevel #0 unset $var
}
}
# Put all exit related code here.
proc ExitProg {} {
exit
}
proc CreateWindow {} {
global g_MyCanv
set w 300
set h 300
set cw [expr $w / 2]
set ch [expr $h / 2]
# Create top frame.
frame .fr
pack .fr -expand 1 -fill both
# Add a canvas and info widgets.
set g_MyCanv .fr.c
canvas $g_MyCanv -bg green
listbox .fr.usage -height 3 -font $::listFont
label .fr.info
grid $g_MyCanv -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: Togl window in canvas"
# Fill the canvas with a togl window and some button widgets.
togl $g_MyCanv.toglwin -width $cw -height $ch \
-swapinterval 1 \
-double true -depth true \
-createcommand CreateCallback \
-displaycommand DisplayCallback \
-reshapecommand ReshapeCallback
$g_MyCanv create window $cw $ch -window $g_MyCanv.toglwin \
-anchor center -tags "TOGLWIN"
button $g_MyCanv.b_se -relief groove -text "SE" -command {ShiftImg "SE"} \
-repeatdelay 200 -repeatinterval 100
$g_MyCanv create window $w $h -window $g_MyCanv.b_se -anchor se -tags "SE"
button $g_MyCanv.b_sw -relief groove -text "SW" -command {ShiftImg "SW"} \
-repeatdelay 200 -repeatinterval 100
$g_MyCanv create window 0 $h -window $g_MyCanv.b_sw -anchor sw -tags "SW"
button $g_MyCanv.b_ne -relief groove -text "NE" -command {ShiftImg "NE"} \
-repeatdelay 200 -repeatinterval 100
$g_MyCanv create window $w 0 -window $g_MyCanv.b_ne -anchor ne -tags "NE"
button $g_MyCanv.b_nw -relief groove -text "NW" -command {ShiftImg "NW"} \
-repeatdelay 200 -repeatinterval 100
$g_MyCanv create window 0 0 -window $g_MyCanv.b_nw -anchor nw -tags "NW"
# Create some canvas text items for printing messages.
$g_MyCanv create text $cw 20 -tags "TEXT1" -anchor center \
-text "This is the canvas background"
$g_MyCanv create text $cw [expr $h -20] -tags "TEXT2" -anchor center \
-text "This is the canvas background"
bind $g_MyCanv <Configure> "ReshapeCanvas .fr.c"
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
bind $g_MyCanv.toglwin <1> "StartAnimation"
bind $g_MyCanv.toglwin <2> "StopAnimation"
bind $g_MyCanv.toglwin <3> "StopAnimation"
bind $g_MyCanv.toglwin <Control-Button-1> "StopAnimation"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Mouse-L|MR Start|Stop animation"
.fr.usage insert end "Button Move Togl window"
.fr.usage configure -state disabled
}
CreateWindow
PrintInfo [tcl3dOglGetInfoString]
if { [file tail [info script]] eq [file tail $::argv0] } {
# If started directly from tclsh or wish, then start animation.
update
StartAnimation
}
|
