Demo 1 of 15 in category Tcl3DSpecificDemos
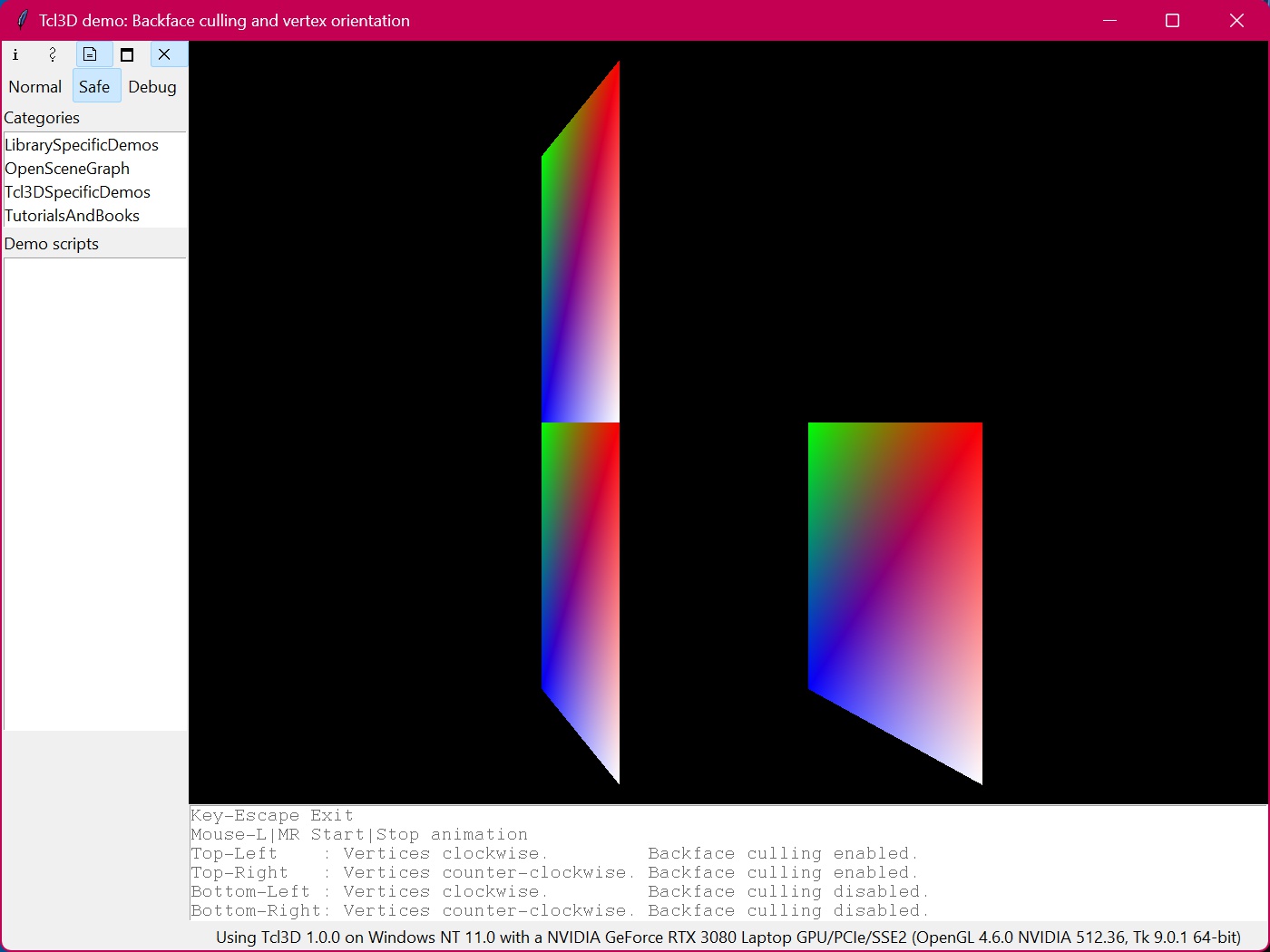 |
# BackfaceCulling.tcl
#
# Demo to show the effects of vertex orientation
# in conjunction with backface culling.
#
# Author: Paul Obermeier
# Date: 2019-05-24
package require tcl3d
# Font to be used in the Tk listbox.
set gDemo(listFont) {-family {Courier} -size 10}
# Window size.
set gDemo(winWidth) 800
set gDemo(winHeight) 600
# Rotation angle for the Quad.
set gDemo(rquad) 0.0
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
ExitProg
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
glViewport 0 0 $w $h
glMatrixMode GL_PROJECTION
glLoadIdentity
gluPerspective 45.0 [expr double($w)/double($h)] 0.1 100.0
glMatrixMode GL_MODELVIEW
glLoadIdentity
set ::gDemo(winWidth) $w
set ::gDemo(winHeight) $h
}
proc CreateCallback { toglwin } {
glShadeModel GL_SMOOTH
glClearColor 0.0 0.0 0.0 0.5
glClearDepth 1.0
glEnable GL_DEPTH_TEST
glDepthFunc GL_LEQUAL
}
proc Animate {} {
.fr.toglwin postredisplay
set ::animateId [tcl3dAfterIdle Animate]
}
proc StartAnimation {} {
if { ! [info exists ::animateId] } {
Animate
}
}
proc StopAnimation {} {
if { [info exists ::animateId] } {
after cancel $::animateId
unset ::animateId
}
}
# Create a Quad by specifying the vertices clockwise.
proc DrawQuadClockwise { tx ty tz angle } {
glLoadIdentity ; # Reset the current Modelview matrix
glTranslatef $tx $ty $tz ; # Translate the Quad
glRotatef $angle 0.0 1.0 0.0 ; # Rotate the Quad on the Y axis
glBegin GL_QUADS
glColor3f 1.0 0.0 0.0 ; # Set the color to red
glVertex3f -1.0 1.0 0.0 ; # Top-Left
glColor3f 0.0 1.0 0.0 ; # Set the color to green
glVertex3f 1.0 1.0 0.0 ; # Top-Right
glColor3f 0.0 0.0 1.0 ; # Set the color to blue
glVertex3f 1.0 -1.0 0.0 ; # Bottom-Right
glColor3f 1.0 1.0 1.0 ; # Set the color to white
glVertex3f -1.0 -1.0 0.0 ; # Bottom-Left
glEnd
}
# Create a Quad by specifying the vertices counter-clockwise.
proc DrawQuadCounterClockwise { tx ty tz angle } {
glLoadIdentity ; # Reset the current Modelview matrix
glTranslatef $tx $ty $tz ; # Translate the Quad
glRotatef $angle 0.0 1.0 0.0 ; # Rotate the Quad on the Y axis
glBegin GL_QUADS
glColor3f 1.0 0.0 0.0 ; # Set the color to red
glVertex3f -1.0 1.0 0.0 ; # Top-Left
glColor3f 1.0 1.0 1.0 ; # Set the color to white
glVertex3f -1.0 -1.0 0.0 ; # Bottom-Left
glColor3f 0.0 0.0 1.0 ; # Set the color to blue
glVertex3f 1.0 -1.0 0.0 ; # Bottom-Right
glColor3f 0.0 1.0 0.0 ; # Set the color to green
glVertex3f 1.0 1.0 0.0 ; # Top-Right
glEnd
}
proc DisplayCallback { toglwin } {
# Clear Color and Depth buffer
glClear [expr $::GL_COLOR_BUFFER_BIT | $::GL_DEPTH_BUFFER_BIT]
# Viewport command is not really needed, but has been inserted for
# Mac OSX. Presentation framework (Tk) does not send a reshape event,
# when switching from one demo to another.
glViewport 0 0 [$toglwin width] [$toglwin height]
# Draw the top 2 Quads with backface culling enabled.
glEnable GL_CULL_FACE
DrawQuadClockwise -1.0 1.0 -6.0 $::gDemo(rquad)
DrawQuadCounterClockwise 1.0 1.0 -6.0 $::gDemo(rquad)
# Draw the lower 2 Quads with backface culling disabled.
glDisable GL_CULL_FACE
DrawQuadClockwise -1.0 -1.0 -6.0 $::gDemo(rquad)
DrawQuadCounterClockwise 1.0 -1.0 -6.0 $::gDemo(rquad)
# Increase the rotation variable for the Quad.
set ::gDemo(rquad) [expr $::gDemo(rquad) + 0.05]
$toglwin swapbuffers
}
# Put all exit related code here.
proc ExitProg {} {
exit
}
# Create the OpenGL window and some Tk helper widgets.
proc CreateWindow {} {
frame .fr
pack .fr -expand 1 -fill both
togl .fr.toglwin -width $::gDemo(winWidth) -height $::gDemo(winHeight) \
-double true -depth true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::gDemo(listFont) -height 6
label .fr.info
grid .fr.toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: Backface culling and vertex orientation"
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
bind .fr.toglwin <1> "StartAnimation"
bind .fr.toglwin <2> "StopAnimation"
bind .fr.toglwin <3> "StopAnimation"
bind .fr.toglwin <Control-Button-1> "StopAnimation"
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Mouse-L|MR Start|Stop animation"
.fr.usage insert end "Top-Left : Vertices clockwise. Backface culling enabled."
.fr.usage insert end "Top-Right : Vertices counter-clockwise. Backface culling enabled."
.fr.usage insert end "Bottom-Left : Vertices clockwise. Backface culling disabled."
.fr.usage insert end "Bottom-Right: Vertices counter-clockwise. Backface culling disabled."
.fr.usage configure -state disabled
}
CreateWindow
PrintInfo [tcl3dOglGetInfoString]
if { [file tail [info script]] eq [file tail $::argv0] } {
# If started directly from tclsh or wish, then start animation.
update
StartAnimation
}
|
