Demo 5 of 9 in category NPS-Tutorials
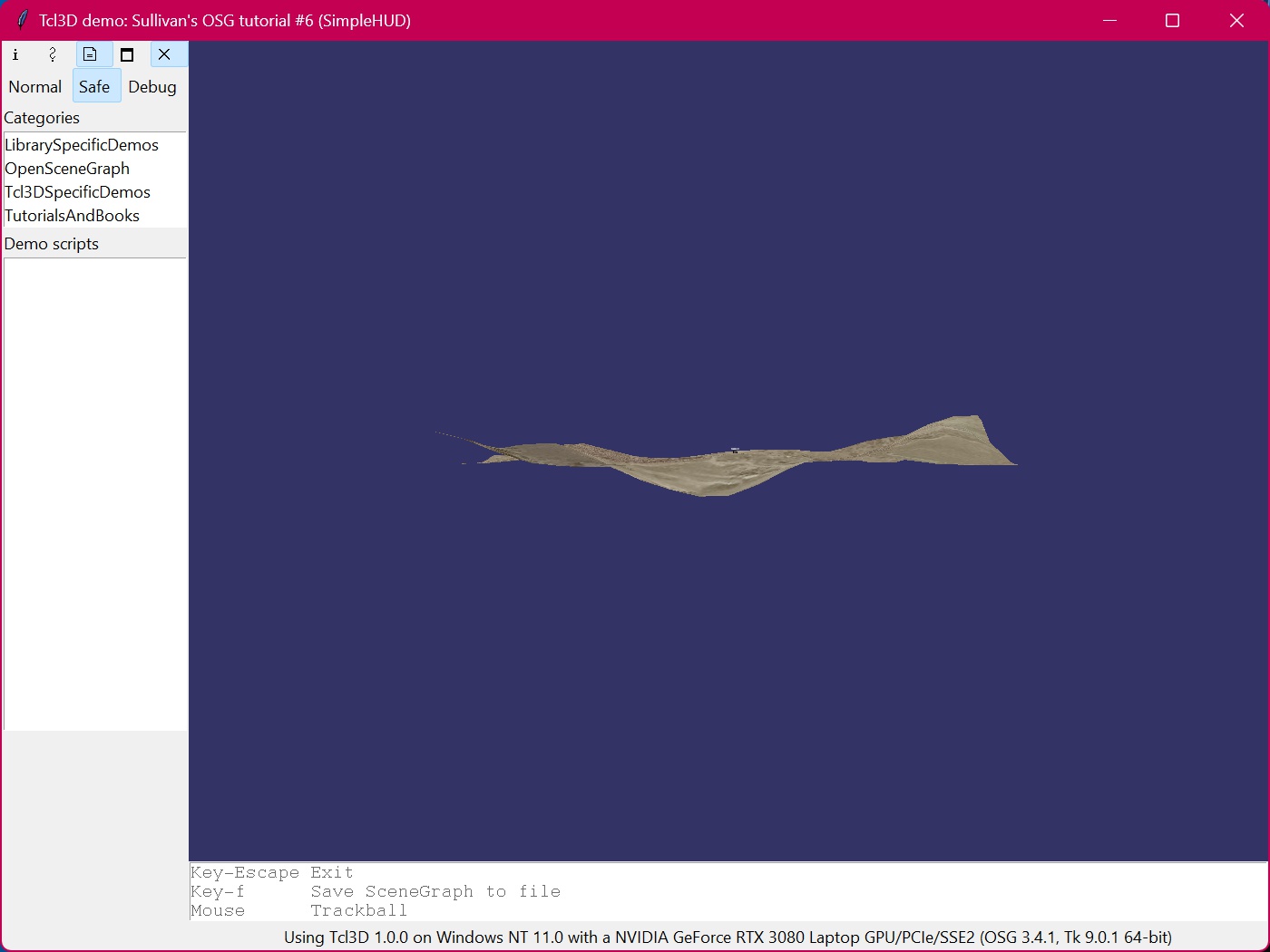 |
# NPS06_SimpleHUD.tcl
#
# Original C++ code by Joseph Sullivan.
# See http://www.openscenegraph.org/projects/osg/wiki/Support/Tutorials
# for the original files.
#
# Modified for Tcl3D by Paul Obermeier 2009/03/20.
# See www.tcl3d.org for the Tcl3D extension.
package require tcl3d
if { ! [tcl3dHaveOsg] } {
tk_messageBox -icon error -type ok -title "Missing Tcl3D module" \
-message "Demo needs the tcl3dOSG module."
proc Cleanup {} {}
exit 1
return
}
# Font to be used in the Tk listbox.
set gDemo(listFont) {-family {Courier} -size 10}
# Window size.
set gDemo(winWidth) 640
set gDemo(winHeight) 480
# Determine the directory and filename of this script.
set gDemo(scriptFile) [info script]
set gDemo(scriptDir) [file dirname $gDemo(scriptFile)]
# Show errors occuring in the Togl callbacks.
proc bgerror { msg } {
puts "Error: $msg\n\n$::errorInfo"
tk_messageBox -icon error -type ok -message "Error: $msg\n\n$::errorInfo"
ExitProg
}
# Print info message into widget a the bottom of the window.
proc PrintInfo { msg } {
if { [winfo exists .fr.info] } {
.fr.info configure -text $msg
}
}
# Idle callback to redisplay the scene.
proc Animate {} {
.fr.toglwin postredisplay
set ::animateId [tcl3dAfterIdle Animate]
}
proc StartAnimation {} {
if { ! [info exists ::animateId] } {
Animate
}
}
proc StopAnimation {} {
if { [info exists ::animateId] } {
after cancel $::animateId
unset ::animateId
}
}
proc CreateCallback { toglwin } {
}
proc ReshapeCallback { toglwin { w -1 } { h -1 } } {
set w [$toglwin width]
set h [$toglwin height]
# Propagate resize event to embedded OSG window.
tcl3dOsgWindowResize $toglwin [tcl3dOsgGetOsgWin] $w $h
}
proc DisplayCallback { toglwin } {
if { [viewer valid] } {
viewer frame
}
$toglwin swapbuffers
}
proc Cleanup {} {
uplevel #0 unset gDemo
viewer -delete
}
proc ExitProg {} {
exit
}
proc SaveOsgToFile {} {
global gDemo
set osgRoot [viewer getSceneData]
set outFile [format "%s.osgt" [file rootname $gDemo(scriptFile)]]
# Create a name on the file system, if running from within a Starpack.
set outFile [tcl3dGenExtName $outFile]
puts "Saving scenegraph to file $outFile"
if { ! [osgDB::writeNodeFile $osgRoot $outFile] } {
puts "Failed to write scenegraph to file $outFile"
}
}
proc CreateWidgets { osgwin } {
global gDemo
frame .fr
pack .fr -expand 1 -fill both
set toglwin .fr.toglwin
togl $toglwin -width $gDemo(winWidth) -height $gDemo(winHeight) \
-double true -depth true -alpha true \
-createcommand CreateCallback \
-reshapecommand ReshapeCallback \
-displaycommand DisplayCallback
listbox .fr.usage -font $::gDemo(listFont) -height 3
label .fr.info
grid $toglwin -row 0 -column 0 -sticky news
grid .fr.usage -row 1 -column 0 -sticky news
grid .fr.info -row 2 -column 0 -sticky news
grid rowconfigure .fr 0 -weight 1
grid columnconfigure .fr 0 -weight 1
wm title . "Tcl3D demo: Sullivan's OSG tutorial #6 (SimpleHUD)"
wm protocol . WM_DELETE_WINDOW "ExitProg"
bind . <Key-Escape> "ExitProg"
bind . <Key-f> "SaveOsgToFile"
# Propagate key and mouse events to embedded OSG window.
bind . <KeyPress> "tcl3dOsgKeyPress $toglwin $osgwin %N"
tcl3dOsgAddTrackballBindings $toglwin $osgwin
.fr.usage insert end "Key-Escape Exit"
.fr.usage insert end "Key-f Save SceneGraph to file"
.fr.usage insert end "Mouse Trackball"
.fr.usage configure -state disabled
}
#
# Start of tutorial specific code.
#
osg::Group root
osg::PositionAttitudeTransform tankXform
osg::Vec3d tankPosit
osg::Geode HUDGeode
osgText::Text textOne
osgText::Text tankLabel
osg::Projection HUDProjectionMatrix
osgViewer::ViewerRef viewer [osgViewer::Viewer]
set tankFile [file join $::gDemo(scriptDir) "Data/Models/t72-tank/t72-tank_des.flt"]
set terrainFile [file join $::gDemo(scriptDir) "Data/Models/JoeDirt/JoeDirt.flt"]
set tankNode [osgDB::readNodeFile $tankFile]
set terrainNode [osgDB::readNodeFile $terrainFile]
tankPosit set 5 5 8
tankXform setPosition tankPosit
root addChild $terrainNode
root addChild tankXform
tankXform addChild $tankNode
HUDProjectionMatrix setMatrix [osg::Matrixd_ortho2D 0 1024 0 768]
osg::MatrixTransform HUDModelViewMatrix
HUDModelViewMatrix setReferenceFrame $::osg::Transform_ABSOLUTE_RF
HUDModelViewMatrix setMatrix [osg::Matrix identity]
root addChild HUDProjectionMatrix
HUDProjectionMatrix addChild HUDModelViewMatrix
HUDModelViewMatrix addChild HUDGeode
HUDGeode addDrawable textOne
textOne setCharacterSize 25
textOne setFont "./Data/impact.ttf"
textOne setText "Not so good"
textOne setAxisAlignment $::osgText::Text_SCREEN
textOne setPosition [osg::Vec3 v1 242 165 1]
textOne setColor [osg::Vec4 v2 1 0 0 1]
tankLabel setCharacterSize 2
tankLabel setFont "./Data/impact.ttf"
tankLabel setText "Tank #1"
tankLabel setAxisAlignment $::osgText::Text_SCREEN
tankLabel setDrawMode [expr $::osgText::Text_TEXT | \
$::osgText::Text_ALIGNMENT | \
$::osgText::Text_BOUNDINGBOX]
tankLabel setAlignment $::osgText::Text_CENTER_TOP
tankLabel setPosition [osg::Vec3 v3 0 0 4]
tankLabel setColor [osg::Vec4 v4 1 1 1 1]
osg::Geode tankLabelGeode
tankLabelGeode addDrawable tankLabel
tankXform addChild tankLabelGeode
osg::Geometry HUDBackgroundGeometry
osg::Vec3Array HUDBackgroundVertices
HUDBackgroundVertices push [osg::Vec3 p1 0 0 0.8]
HUDBackgroundVertices push [osg::Vec3 p2 1024 0 0.8]
HUDBackgroundVertices push [osg::Vec3 p3 1024 200 0.8]
HUDBackgroundVertices push [osg::Vec3 p4 0 200 0.8]
osg::DrawElementsUInt HUDBackgroundIndices $::osg::PrimitiveSet_POLYGON 0
HUDBackgroundIndices push 0
HUDBackgroundIndices push 1
HUDBackgroundIndices push 2
HUDBackgroundIndices push 3
osg::Vec4Array HUDcolors
HUDcolors push [osg::Vec4 c1 0.8 0.8 0.8 0.5]
osg::Vec2Array texcoords 4
texcoords set 0 [osg::Vec2 v1 0.0 0.0]
texcoords set 1 [osg::Vec2 v2 1.0 0.0]
texcoords set 2 [osg::Vec2 v3 1.0 1.0]
texcoords set 3 [osg::Vec2 v4 0.0 1.0]
HUDBackgroundGeometry setTexCoordArray 0 texcoords
# set up the texture state.
osg::Texture2D HUDTexture
# protect from being optimized away as static state.
HUDTexture setDataVariance $::osg::Object_DYNAMIC
HUDTexture setImage [osgDB::readImageFile "./Data/Textures/sky.bmp"]
osg::Vec3Array HUDnormals
HUDnormals push [osg::Vec3 h1 0.0 0.0 1.0]
HUDBackgroundGeometry setNormalArray HUDnormals
HUDBackgroundGeometry setNormalBinding $::osg::Geometry_BIND_OVERALL
HUDGeode addDrawable HUDBackgroundGeometry
HUDBackgroundGeometry addPrimitiveSet HUDBackgroundIndices
HUDBackgroundGeometry setVertexArray HUDBackgroundVertices
HUDBackgroundGeometry setColorArray HUDcolors
HUDBackgroundGeometry setColorBinding $::osg::Geometry_BIND_OVERALL
osg::StateSet HUDStateSet
HUDGeode setStateSet HUDStateSet
HUDStateSet setRenderBinDetails 11 "DepthSortedBin"
HUDStateSet setMode $GL_BLEND $::osg::StateAttribute_ON
HUDStateSet setMode $GL_DEPTH_TEST $::osg::StateAttribute_OFF
HUDStateSet setRenderingHint $::osg::StateSet_TRANSPARENT_BIN
HUDStateSet setTextureAttributeAndModes 0 HUDTexture $::osg::StateAttribute_ON
osgUtil::RenderBin HUDBin $::osgUtil::RenderBin_SORT_BACK_TO_FRONT
viewer setSceneData root
viewer setCameraManipulator [osgGA::TrackballManipulator]
if { $argc >= 1 && [lindex $argv 0] eq "-viewer" } {
# Only use the standard OSG viewer window without any Tk widgets.
viewer setUpViewInWindow 50 50 500 400
viewer run
exit 0
}
# Use the OSG viewer inside a Togl widget.
set osgwin [viewer setUpViewerAsEmbeddedInWindow 50 50 500 400]
tcl3dOsgSetOsgWin $osgwin
viewer realize
CreateWidgets $osgwin
PrintInfo [tcl3dOsgGetInfoString]
if { [file tail [info script]] eq [file tail $::argv0] } {
# If started directly from tclsh or wish, then start animation.
update
StartAnimation
}
|
